Reactstrap Images
Documentation and examples for opting images into responsive behavior (so they never become larger than their parent elements) and add lightweight styles to them—all via classes.
Responsive Images
Images in Bootstrap are made responsive with
.img-fluid
, having
max-width: 100%;
and
height: auto;
are applied to the image so that it scales with the parent element.
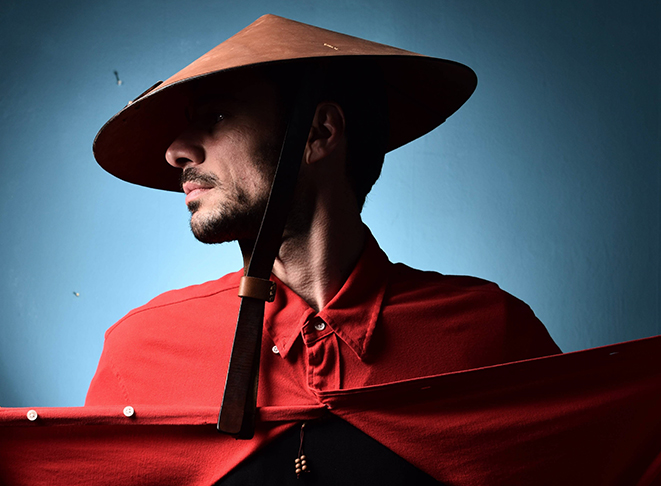
import React from "react";
// reactstrap components
// import {
//
// } from "reactstrap";
function Example() {
return (
<>
<img
src="https://demos.creative-tim.com/argon-design-system-pro/assets/img/faces/team-5.jpg"
className="img-fluid"
alt="..."
/>
</>
);
}
export default Example;
SVG images and IE 10
In Internet Explorer 10, SVG images with
.img-fluid
are disproportionately sized. To fix this, add
width: 100% \9;
where necessary. This fix improperly sizes other image formats, so
Bootstrap doesn’t apply it automatically.
Image thumbnails
In addition to
border-radius utilities, you can use
.img-thumbnail
to give an image a rounded 1px border appearance.
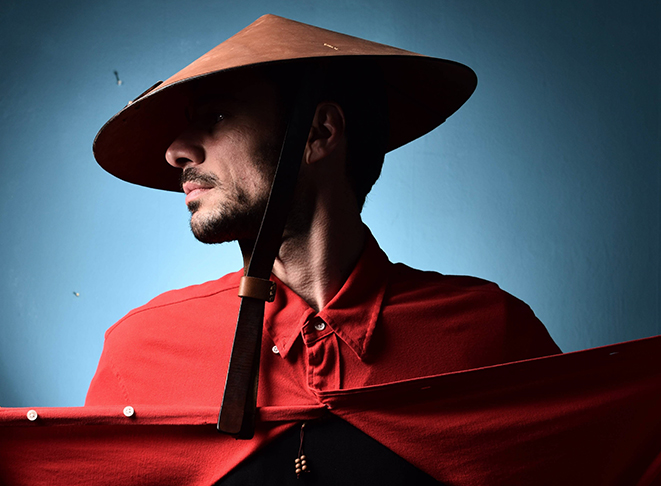
import React from "react";
// reactstrap components
// import {
//
// } from "reactstrap";
function Example() {
return (
<>
<img
src="https://demos.creative-tim.com/argon-design-system-pro/assets/img/faces/team-5.jpg"
className="img-thumbnail"
alt="A generic square placeholder with a white border around it, making it resemble a photograph taken with an old instant camera"
/>
</>
);
}
export default Example;
Examples
import React from "react";
// reactstrap components
import { Row, Col, UncontrolledTooltip } from "reactstrap";
const style = { width: "150px" };
function Example() {
return (
<>
<Row>
<Col sm="3" xs="6">
<small className=" d-block text-uppercase font-weight-bold mb-4">
Image
</small>
<img
alt="..."
className=" img-fluid rounded shadow"
src="https://demos.creative-tim.com/argon-design-system-pro/assets/img/faces/team-1.jpg"
style={style}
></img>
</Col>
<Col sm="3" xs="6">
<small className=" d-block text-uppercase font-weight-bold mb-4">
Circle Image
</small>
<img
alt="..."
className=" img-fluid rounded-circle shadow"
src="https://demos.creative-tim.com/argon-design-system-pro/assets/img/faces/team-2.jpg"
style={ style }
></img>
</Col>
<Col className=" mt-5 mt-sm-0" sm="3" xs="6">
<small className=" d-block text-uppercase font-weight-bold mb-4">
Raised
</small>
<img
alt="..."
className=" img-fluid rounded shadow-lg"
src="https://demos.creative-tim.com/argon-design-system-pro/assets/img/faces/team-3.jpg"
style={ style }
></img>
</Col>
<Col className=" mt-5 mt-sm-0" sm="3" xs="6">
<small className=" d-block text-uppercase font-weight-bold mb-4">
Circle Raised
</small>
<img
alt="..."
className=" img-fluid rounded-circle shadow-lg"
src="https://demos.creative-tim.com/argon-design-system-pro/assets/img/faces/team-4.jpg"
style={ style }
></img>
</Col>
</Row>
<Row className=" mt-5">
<Col sm="3" xs="6">
<small className=" d-block text-uppercase font-weight-bold mb-4">
Avatar group
</small>
<div className=" avatar-group">
<a
className=" avatar avatar-lg rounded-circle"
href="#pablo"
onClick={(e) => e.preventDefault()}
id="tooltip475708683"
>
<img
alt="..."
src="https://demos.creative-tim.com/argon-design-system-pro/assets/img/faces/team-1.jpg"
></img>
</a>
<UncontrolledTooltip delay={0} target="tooltip475708683">
Ryan Tompson
</UncontrolledTooltip>
<a
className=" avatar avatar-lg rounded-circle"
href="#pablo"
onClick={(e) => e.preventDefault()}
id="tooltip138001378"
>
<img
alt="..."
src="https://demos.creative-tim.com/argon-design-system-pro/assets/img/faces/team-2.jpg"
></img>
</a>
<UncontrolledTooltip delay={0} target="tooltip138001378">
Romina Hadid
</UncontrolledTooltip>
<a
className=" avatar avatar-lg rounded-circle"
href="#pablo"
onClick={(e) => e.preventDefault()}
id="tooltip399019999"
>
<img
alt="..."
src="https://demos.creative-tim.com/argon-design-system-pro/assets/img/faces/team-3.jpg"
></img>
</a>
<UncontrolledTooltip delay={0} target="tooltip399019999">
Alexander Smith
</UncontrolledTooltip>
<a
className=" avatar avatar-lg rounded-circle"
href="#pablo"
onClick={(e) => e.preventDefault()}
id="tooltip702156286"
>
<img
alt="..."
src="https://demos.creative-tim.com/argon-design-system-pro/assets/img/faces/team-4.jpg"
></img>
</a>
<UncontrolledTooltip delay={0} target="tooltip702156286">
Jessica Doe
</UncontrolledTooltip>
</div>
</Col>
<Col sm="6" xs="6">
<small className=" d-block text-uppercase font-weight-bold mb-4">
Sizing
</small>
<a
className=" avatar avatar-xs rounded-circle"
href="#pablo"
onClick={(e) => e.preventDefault()}
>
<img
alt="..."
src="https://demos.creative-tim.com/argon-design-system-pro/assets/img/faces/team-4.jpg"
></img>
</a>
<a
className=" avatar avatar-sm rounded-circle"
href="#pablo"
onClick={(e) => e.preventDefault()}
>
<img
alt="..."
src="https://demos.creative-tim.com/argon-design-system-pro/assets/img/faces/team-4.jpg"
></img>
</a>
<a
className=" avatar rounded-circle"
href="#pablo"
onClick={(e) => e.preventDefault()}
>
<img
alt="..."
src="https://demos.creative-tim.com/argon-design-system-pro/assets/img/faces/team-4.jpg"
></img>
</a>
<a
className=" avatar avatar-lg rounded-circle"
href="#pablo"
onClick={(e) => e.preventDefault()}
>
<img
alt="..."
src="https://demos.creative-tim.com/argon-design-system-pro/assets/img/faces/team-4.jpg"
></img>
</a>
<a
className=" avatar avatar-xl rounded-circle"
href="#pablo"
onClick={(e) => e.preventDefault()}
>
<img
alt="..."
src="https://demos.creative-tim.com/argon-design-system-pro/assets/img/faces/team-4.jpg"
></img>
</a>
</Col>
</Row>
</>
);
}
export default Example;
Aligning images
Align images with the
helper float classes
or
text alignment classes.
block-level
images can be centered using the
.mx-auto
margin utility class.
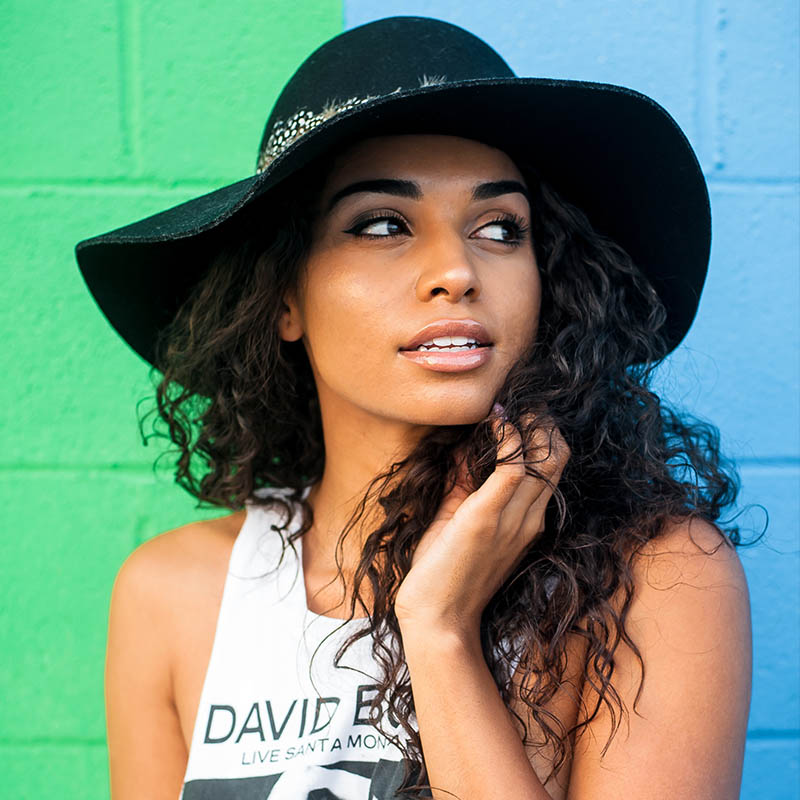
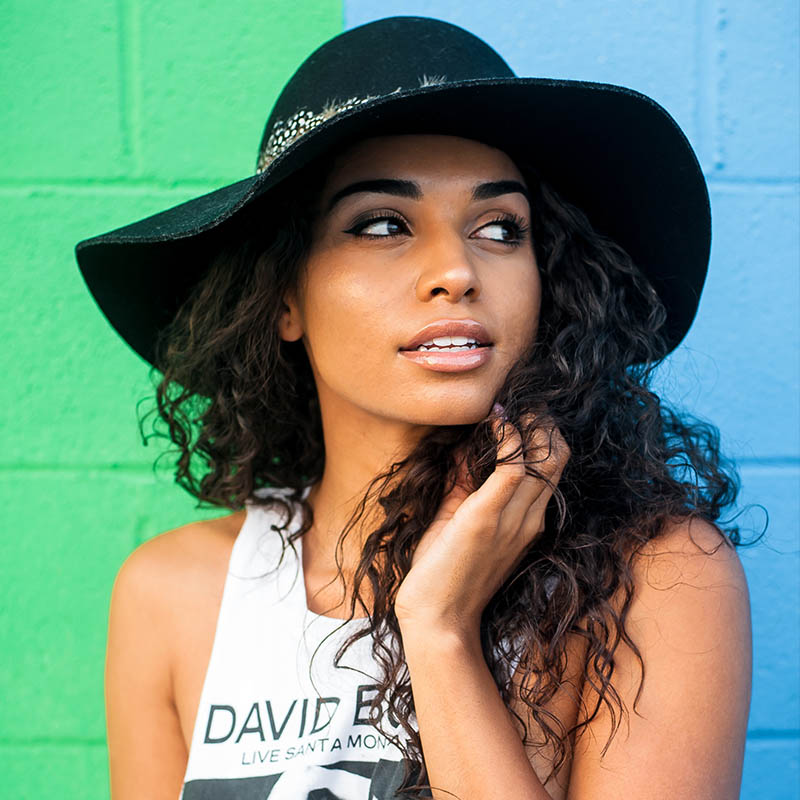
import React from "react";
// reactstrap components
// import {
//
// } from "reactstrap";
const style = { width: "150px" };
function Example() {
return (
<>
<img
src="https://demos.creative-tim.com/argon-design-system-pro/assets/img/faces/team-4.jpg"
className="rounded float-left"
alt="A generic square placeholder with rounded corners"
style={ style }
/>
<img
src="https://demos.creative-tim.com/argon-design-system-pro/assets/img/faces/team-4.jpg"
className="rounded float-right"
alt="A generic square placeholder with rounded corners"
style={ style }
/>
</>
);
}
export default Example;
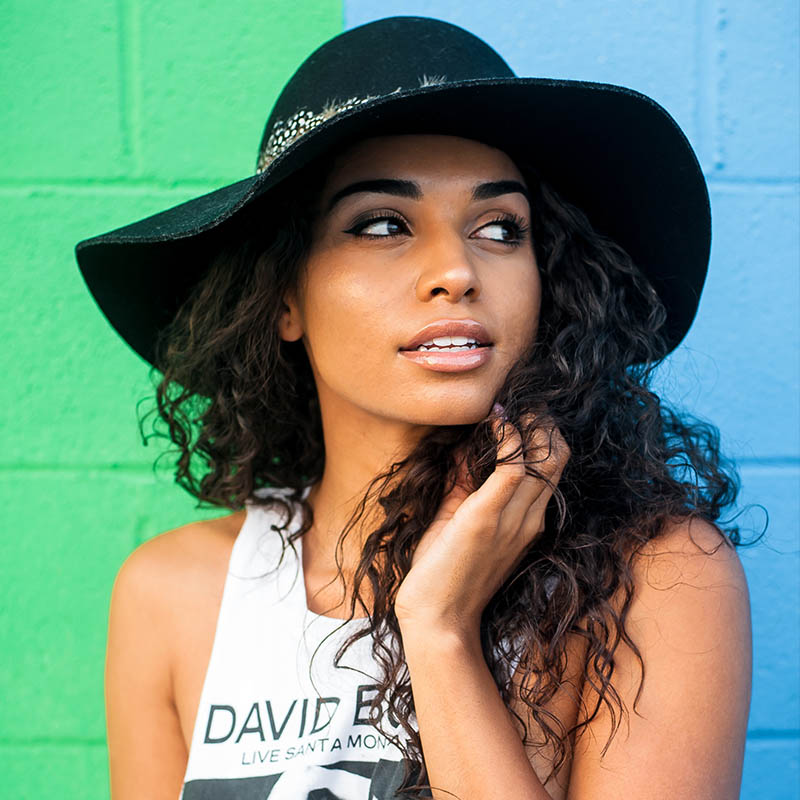
import React from "react";
// reactstrap components
// import {
//
// } from "reactstrap";
const style = { width: "150px" };
function Example() {
return (
<>
<img
src="https://demos.creative-tim.com/argon-design-system-pro/assets/img/faces/team-4.jpg"
className="rounded mx-auto d-block"
style={ style }
alt="A generic square placeholder with rounded corners"
/>
</>
);
}
export default Example;
Picture
If you are using the
<picture>
element to specify multiple
<source>
elements for a specific
<img>
, make sure to add the
.img-*
classes to the
<img>
and not to the
<picture>
tag.
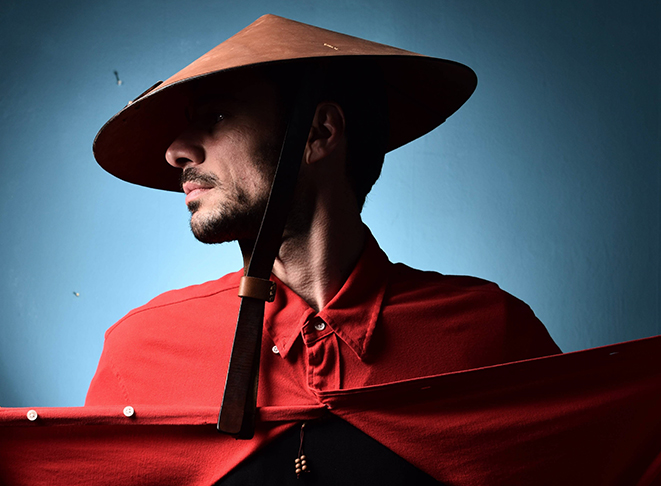
import React from "react";
// reactstrap components
// import {
//
// } from "reactstrap";
const style = { width: "150px" };
function Example() {
return (
<>
<picture>
<img
src="https://demos.creative-tim.com/argon-design-system-pro/assets/img/faces/team-5.jpg"
alt="Rounded "
className="img-fluid rounded shadow"
style={ style }
/>
</picture>
</>
);
}
export default Example;