Nextjs Cards
Our Nextjs cards provide a flexible and extensible content container with multiple variants and options.
Examples
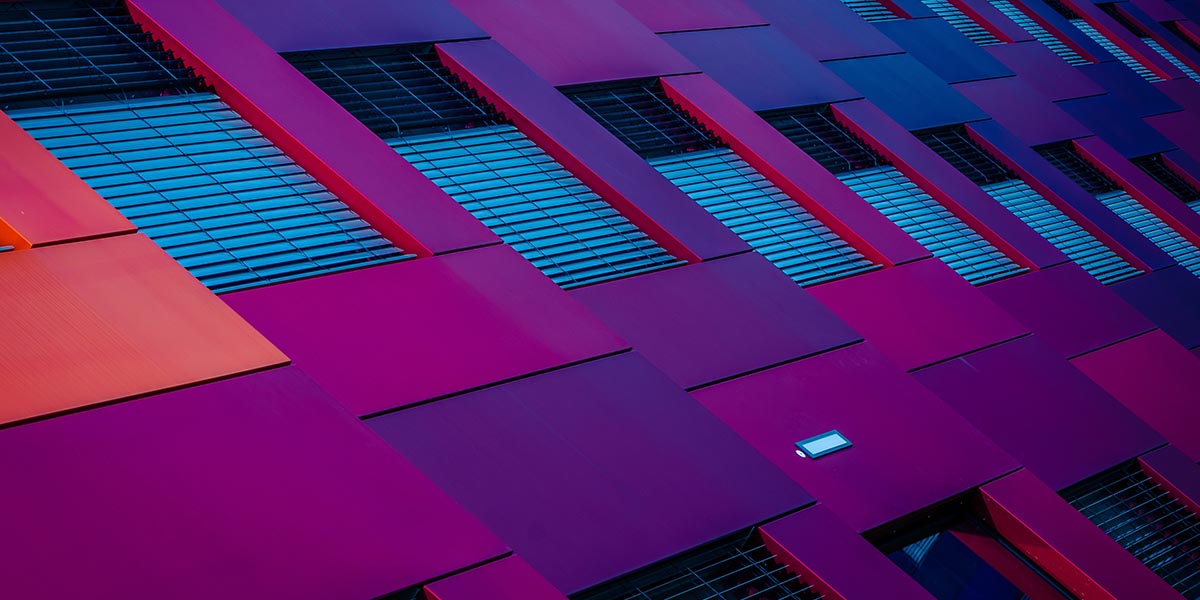
Card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Go somewhereimport React from "react";
// reactstrap components
import {
Button,
Card,
CardBody,
CardImg,
CardTitle,
CardText,
} from "reactstrap";
const cardStyle = { width: "18rem" };
function Example() {
return (
<>
<Card style={cardStyle}>
<CardImg
alt="..."
src={require("assets/img/theme/img-1-1000x600.jpg")}
top
></CardImg>
<CardBody>
<CardTitle>Card title</CardTitle>
<CardText>
Some quick example text to build on the card title and make up the
bulk of the card's content.
</CardText>
<Button color="primary" href="javascript:;">
Go somewhere
</Button>
</CardBody>
</Card>
</>
);
}
export default Example;
Stats card
New users
2,3563.48% Since last month
import React from "react";
// reactstrap components
import { Card, CardBody, CardTitle, Row, Col } from "reactstrap";
const cardStyle = { width: "18rem" };
function Example() {
return (
<>
<div style={cardStyle}>
<Card className="card-stats">
<CardBody>
<Row>
<div className="col">
<CardTitle className="text-uppercase text-muted mb-0">
New users
</CardTitle>
<span className="h2 font-weight-bold mb-0">2,356</span>
</div>
<Col className="col-auto">
<div className="icon icon-shape bg-orange text-white rounded-circle shadow">
<i className="ni ni-chart-pie-35"></i>
</div>
</Col>
</Row>
<p className="mt-3 mb-0 text-sm">
<span className="text-success mr-2">
<i className="fa fa-arrow-up"></i>
3.48%
</span>
<span className="text-nowrap">Since last month</span>
</p>
</CardBody>
</Card>
</div>
</>
);
}
export default Example;
Cards support a wide variety of content, including images, text, list groups, links, and more. Below are examples of what’s supported.
import React from "react";
// reactstrap components
import { Card, CardBody } from "reactstrap";
function Example() {
return (
<>
<Card className="card-frame">
<CardBody>This is some text within a card body.</CardBody>
</Card>
</>
);
}
export default Example;
Layouts
In addition to styling the content within cards, Bootstrap includes a few options for laying out series of cards. For the time being, these layout options are not yet responsive.
Card groups PRO
Need a set of equal width and height cards that aren’t attached to one another? Use card decks.
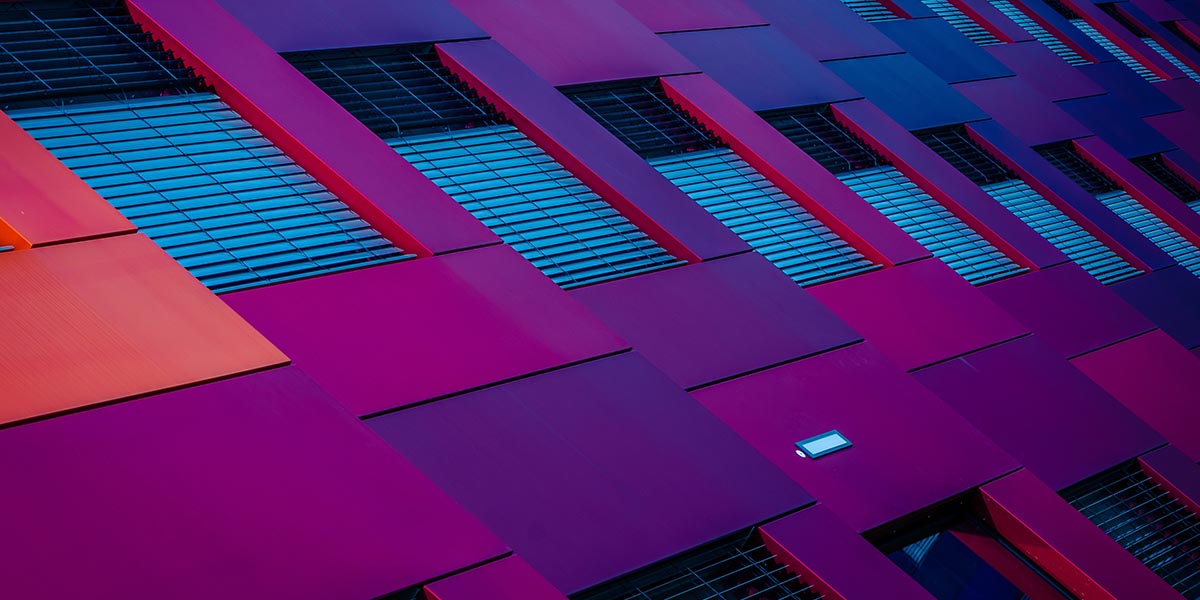
Card title
This is a wider card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
Last updated 3 mins ago
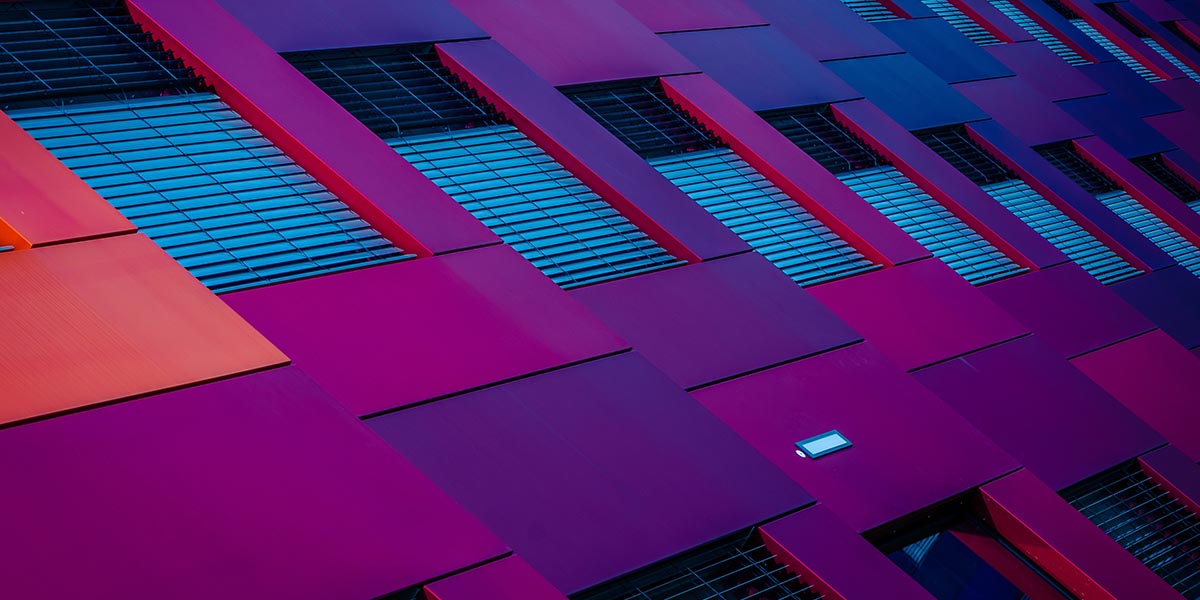
Card title
This card has supporting text below as a natural lead-in to additional content.
Last updated 3 mins ago
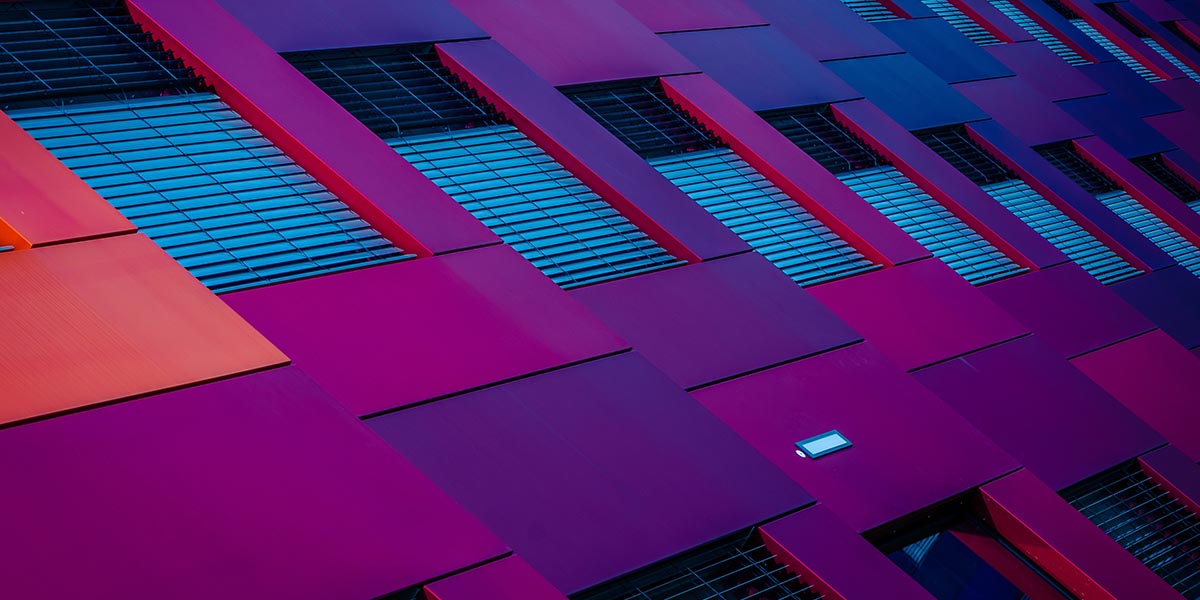
Card title
This is a wider card with supporting text below as a natural lead-in to additional content. This card has even longer content than the first to show that equal height action.
Last updated 3 mins ago
import React from "react";
// reactstrap components
import { CardGroup, Card, CardBody, CardImg, CardTitle, CardText } from "reactstrap";
function Example() {
return (
<>
<CardGroup>
<Card>
<CardImg
alt="..."
src={require("assets/img/theme/img-1-1000x600.jpg")}
top
></CardImg>
<CardBody>
<CardTitle>Card title</CardTitle>
<CardText>
This is a wider card with supporting text below as a natural
lead-in to additional content. This content is a little bit
longer.
</CardText>
<CardText>
<small className="text-muted">Last updated 3 mins ago</small>
</CardText>
</CardBody>
</Card>
<Card>
<CardImg
alt="..."
src={require("assets/img/theme/img-1-1000x600.jpg")}
top
></CardImg>
<CardBody>
<CardTitle>Card title</CardTitle>
<CardText>
This card has supporting text below as a natural lead-in to
additional content.
</CardText>
<CardText>
<small className="text-muted">Last updated 3 mins ago</small>
</CardText>
</CardBody>
</Card>
<Card>
<CardImg
alt="..."
src={require("assets/img/theme/img-1-1000x600.jpg")}
top
></CardImg>
<CardBody>
<CardTitle>Card title</CardTitle>
<CardText>
This is a wider card with supporting text below as a natural
lead-in to additional content. This card has even longer content
than the first to show that equal height action.
</CardText>
<CardText>
<small className="text-muted">Last updated 3 mins ago</small>
</CardText>
</CardBody>
</Card>
</CardGroup>
</>
);
}
export default Example;
Card decks PRO
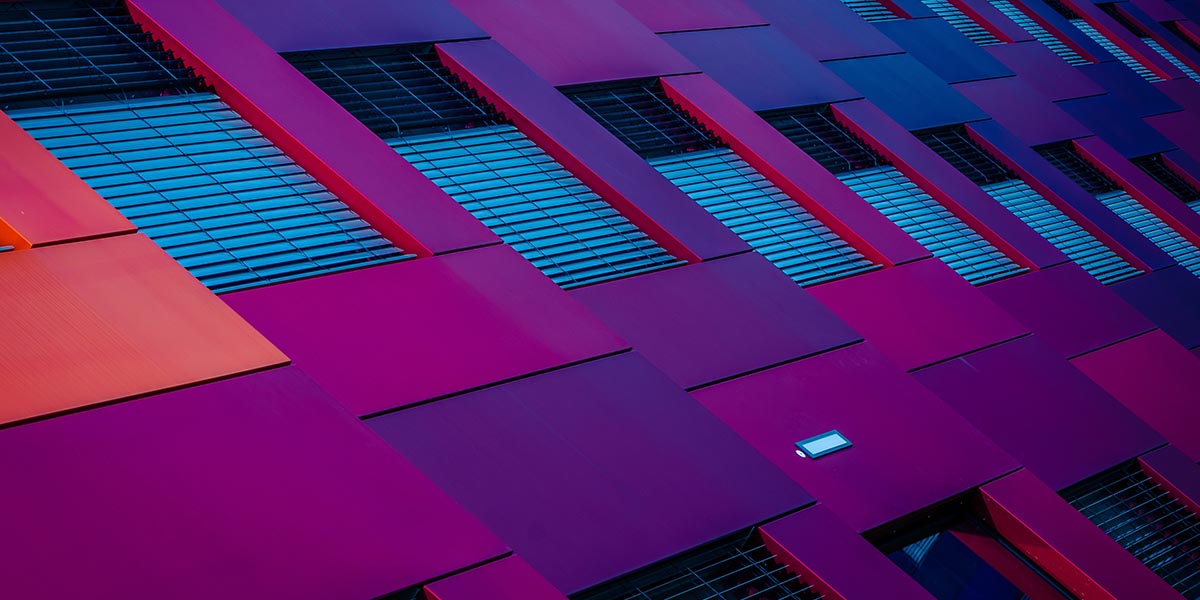
Card title
This is a wider card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
Last updated 3 mins ago
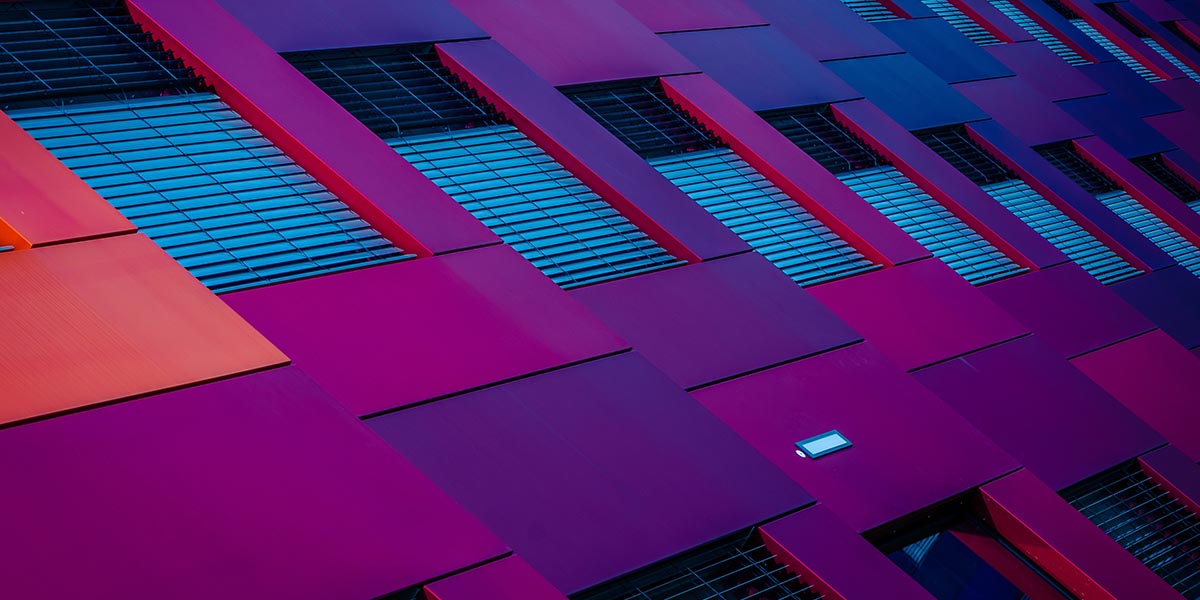
Card title
This card has supporting text below as a natural lead-in to additional content.
Last updated 3 mins ago
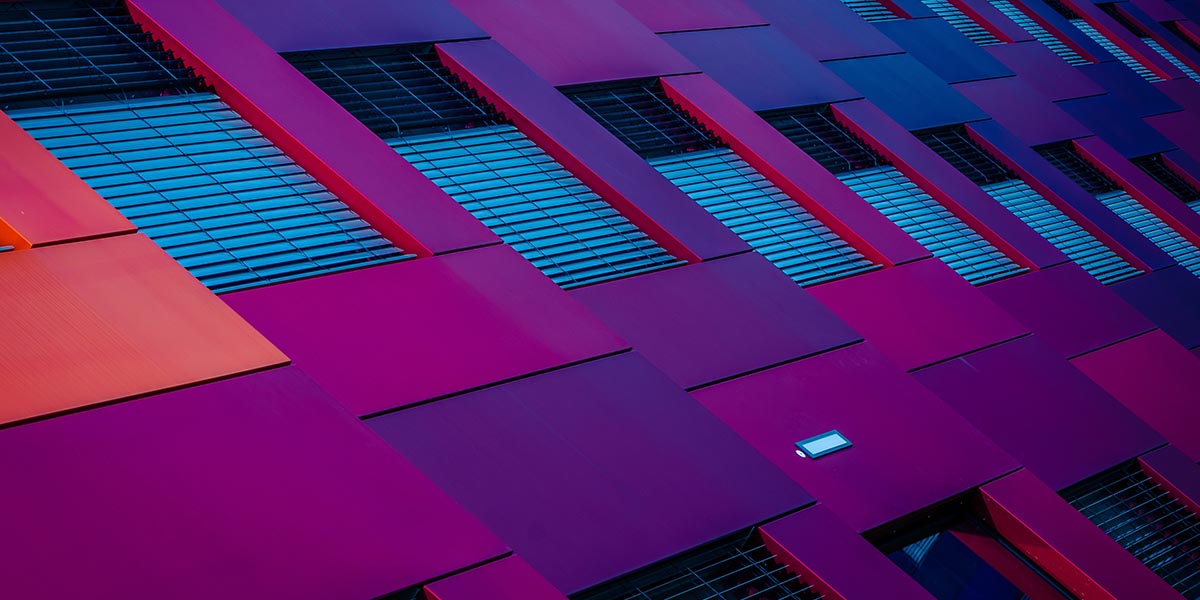
Card title
This is a wider card with supporting text below as a natural lead-in to additional content. This card has even longer content than the first to show that equal height action.
Last updated 3 mins ago
import React from "react";
// reactstrap components
import {
CardDeck,
Card,
CardBody,
CardImg,
CardTitle,
CardText,
} from "reactstrap";
function Example() {
return (
<>
<CardDeck>
<Card>
<CardImg
alt="..."
src={require("assets/img/theme/img-1-1000x600.jpg")}
top
></CardImg>
<CardBody>
<CardTitle>Card title</CardTitle>
<CardText>
This is a wider card with supporting text below as a natural
lead-in to additional content. This content is a little bit
longer.
</CardText>
<CardText>
<small className="text-muted">Last updated 3 mins ago</small>
</CardText>
</CardBody>
</Card>
<Card>
<CardImg
alt="..."
src={require("assets/img/theme/img-1-1000x600.jpg")}
top
></CardImg>
<CardBody>
<CardTitle>Card title</CardTitle>
<CardText>
This card has supporting text below as a natural lead-in to
additional content.
</CardText>
<CardText>
<small className="text-muted">Last updated 3 mins ago</small>
</CardText>
</CardBody>
</Card>
<Card>
<CardImg
alt="..."
src={require("assets/img/theme/img-1-1000x600.jpg")}
top
></CardImg>
<CardBody>
<CardTitle>Card title</CardTitle>
<CardText>
This is a wider card with supporting text below as a natural
lead-in to additional content. This card has even longer content
than the first to show that equal height action.
</CardText>
<CardText>
<small className="text-muted">Last updated 3 mins ago</small>
</CardText>
</CardBody>
</Card>
</CardDeck>
</>
);
}
export default Example;
Card columns PRO
Cards can be organized into Masonry-like columns with just CSS by wrapping them in .card-columns. Cards are built with CSS column properties instead of flexbox for easier alignment. Cards are ordered from top to bottom and left to right.
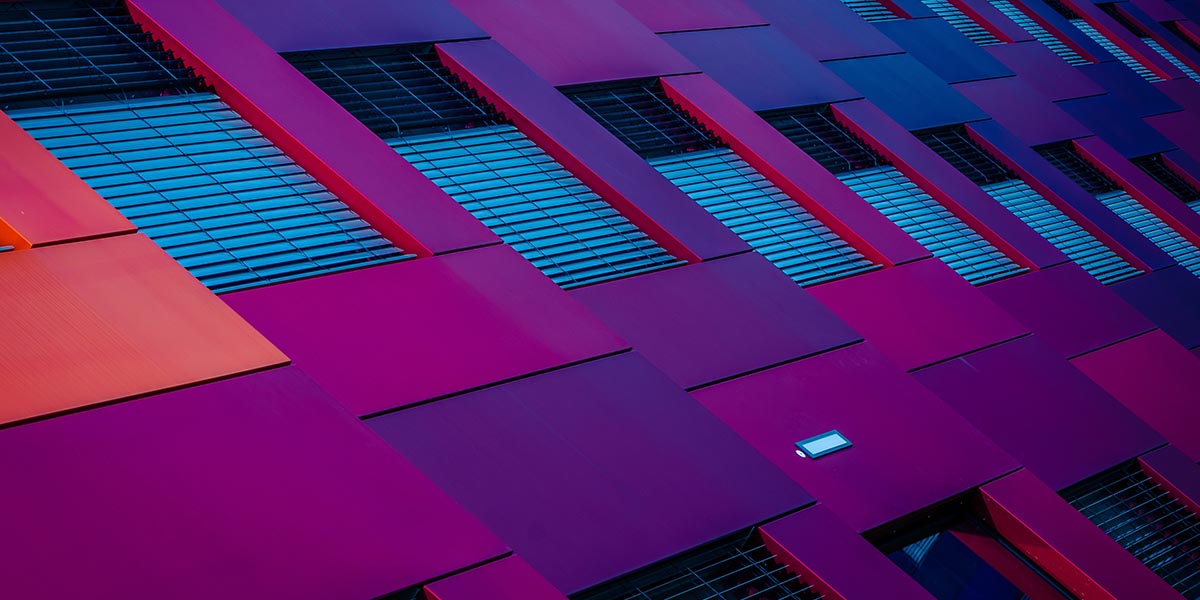
Card title that wraps to a new line
This is a longer card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Integer posuere erat a ante.
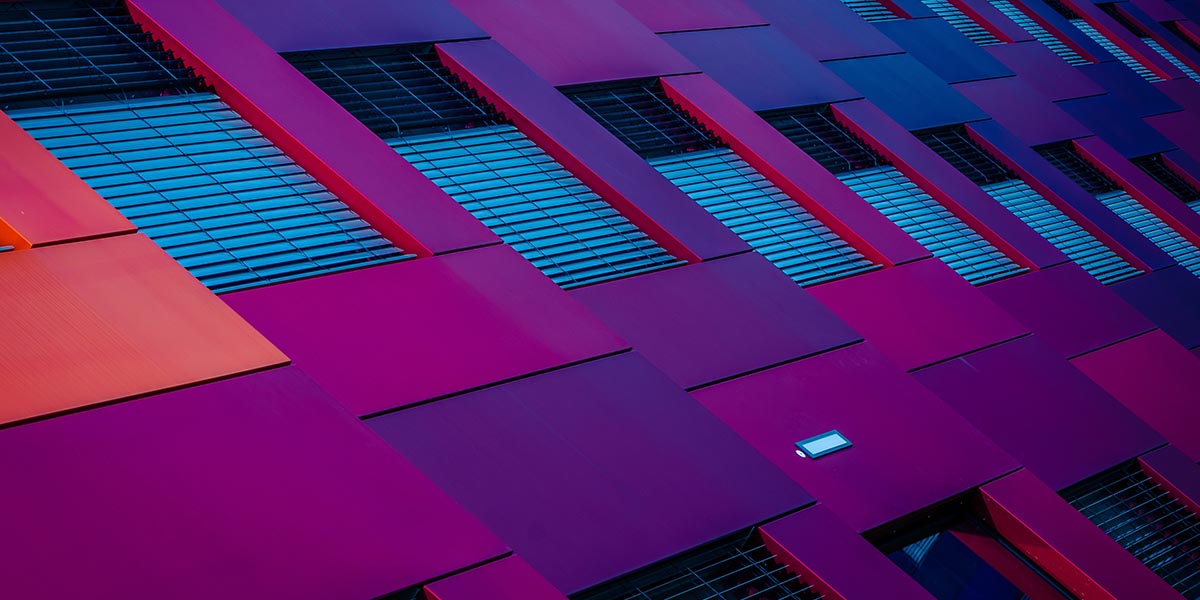
Card title
This card has supporting text below as a natural lead-in to additional content.
Last updated 3 mins ago
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Integer posuere erat.
Card title
This card has a regular title and short paragraphy of text below it.
Last updated 3 mins ago
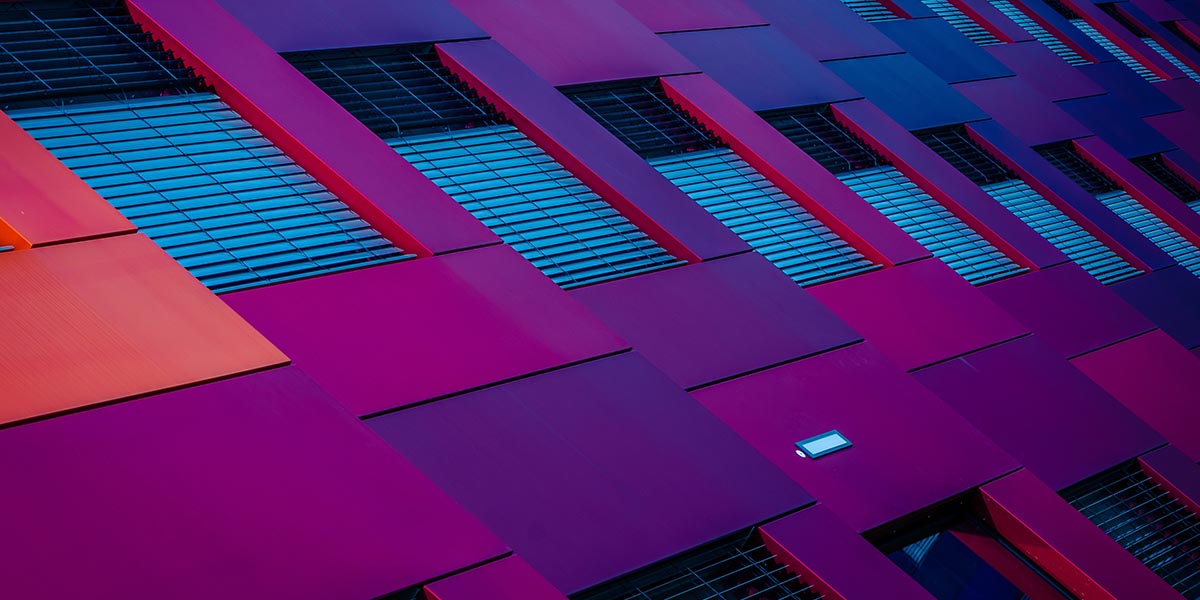
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Integer posuere erat a ante.
Card title
This is another card with title and supporting text below. This card has some additional content to make it slightly taller overall.
Last updated 3 mins ago
import React from "react";
// reactstrap components
import {
CardColumns,
Card,
CardBody,
CardImg,
CardTitle,
CardText,
} from "reactstrap";
function Example() {
return (
<>
<CardColumns>
<Card>
<CardImg
alt="..."
src={require("assets/img/theme/img-1-1000x600.jpg")}
top
></CardImg>
<CardBody>
<CardTitle>Card title that wraps to a new line</CardTitle>
<CardText>
This is a longer card with supporting text below as a natural
lead-in to additional content. This content is a little bit
longer.
</CardText>
</CardBody>
</Card>
<Card className="p-3">
<CardBody className="blockquote mb-0">
<p>
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Integer
posuere erat a ante.
</p>
<footer className="blockquote-footer">
<small className="text-muted">
Someone famous in <cite title="Source Title">Source Title</cite>
</small>
</footer>
</CardBody>
</Card>
<Card>
<CardImg
alt="..."
src={require("assets/img/theme/img-1-1000x600.jpg")}
top
></CardImg>
<CardBody>
<CardTitle>Card title</CardTitle>
<CardText>
This card has supporting text below as a natural lead-in to
additional content.
</CardText>
<CardText>
<small className="text-muted">Last updated 3 mins ago</small>
</CardText>
</CardBody>
</Card>
<Card className="bg-primary text-white text-center p-3">
<blockquote className="blockquote mb-0">
<p>
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Integer
posuere erat.
</p>
<footer className="blockquote-footer">
<small>
Someone famous in <cite title="Source Title">Source Title</cite>
</small>
</footer>
</blockquote>
</Card>
<Card className="text-center">
<CardBody>
<CardTitle>Card title</CardTitle>
<CardText>
This card has a regular title and short paragraphy of text below
it.
</CardText>
<CardText>
<small className="text-muted">Last updated 3 mins ago</small>
</CardText>
</CardBody>
</Card>
<Card>
<CardImg
alt="..."
src={require("assets/img/theme/img-1-1000x600.jpg")}
></CardImg>
</Card>
<Card className="p-3 text-right">
<blockquote className="blockquote mb-0">
<p>
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Integer
posuere erat a ante.
</p>
<footer className="blockquote-footer">
<small className="text-muted">
Someone famous in <cite title="Source Title">Source Title</cite>
</small>
</footer>
</blockquote>
</Card>
<Card>
<CardBody>
<CardTitle>Card title</CardTitle>
<CardText>
This is another card with title and supporting text below. This
card has some additional content to make it slightly taller
overall.
</CardText>
<CardText>
<small className="text-muted">Last updated 3 mins ago</small>
</CardText>
</CardBody>
</Card>
</CardColumns>
</>
);
}
export default Example;
Advanced Examples
List group PRO
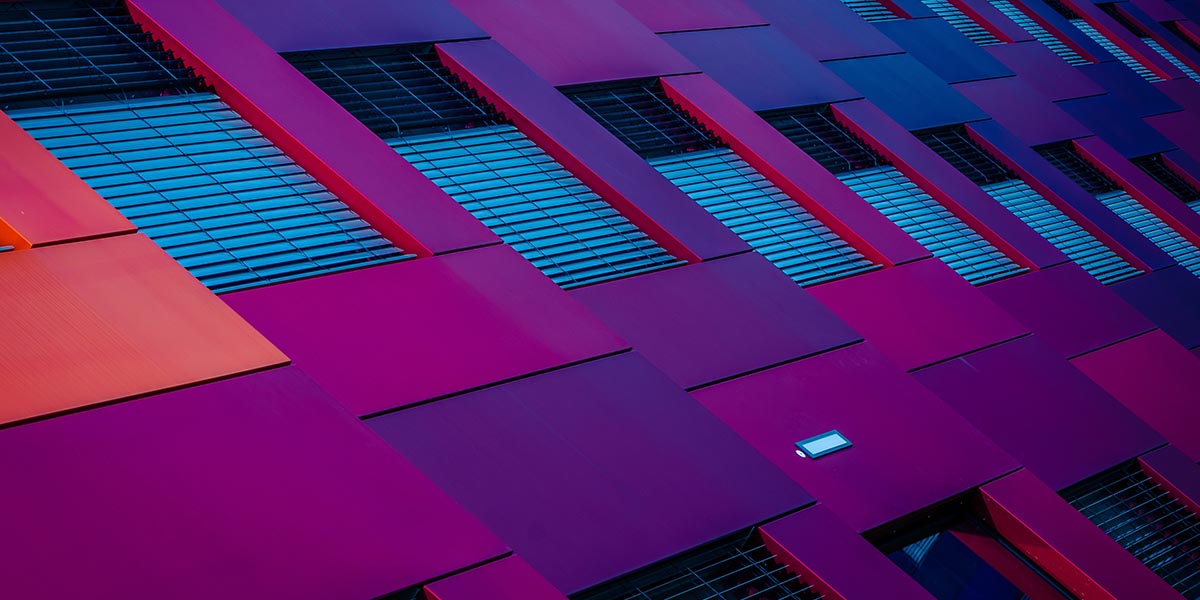
- Cras justo odio
- Dapibus ac facilisis in
- Vestibulum at eros
Card title
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Facilis non dolore est fuga nobis ipsum illum eligendi nemo iure repellat, soluta, optio minus ut reiciendis voluptates enim impedit veritatis officiis.
Go somewhereimport React from "react";
// reactstrap components
import {
Button,
Card,
CardBody,
CardImg,
CardTitle,
CardText,
ListGroupItem,
ListGroup,
Row,
Col,
} from "reactstrap";
function Example() {
return (
<>
<Row>
<Col md="8">
<Card>
<CardImg
alt="..."
src={require("assets/img/theme/img-1-1000x600.jpg")}
top
></CardImg>
<ListGroup flush>
<ListGroupItem>Cras justo odio</ListGroupItem>
<ListGroupItem>Dapibus ac facilisis in</ListGroupItem>
<ListGroupItem>Vestibulum at eros</ListGroupItem>
</ListGroup>
<CardBody>
<CardTitle className="mb-3" tag="h3">
Card title
</CardTitle>
<CardText className="mb-4">
Lorem ipsum dolor sit amet, consectetur adipisicing elit.
Facilis non dolore est fuga nobis ipsum illum eligendi nemo iure
repellat, soluta, optio minus ut reiciendis voluptates enim
impedit veritatis officiis.
</CardText>
<Button color="primary" href="javascript:;">
Go somewhere
</Button>
</CardBody>
</Card>
</Col>
</Row>
</>
);
}
export default Example;
Profile PRO
import React from "react";
// reactstrap components
import {
Button,
Card,
CardHeader,
CardBody,
CardImg,
Row,
Col,
} from "reactstrap";
function Example() {
return (
<>
<Row>
<Col md="6">
<Card className="card-profile">
<CardImg
alt="..."
src={require("assets/img/theme/img-1-1000x600.jpg")}
top
></CardImg>
<Row className="justify-content-center">
<Col className="order-lg-2" lg="3">
<div className="card-profile-image">
<a href="javascript:;">
<img
alt="..."
className="rounded-circle"
src={require("assets/img/theme/team-4.jpg")}
></img>
</a>
</div>
</Col>
</Row>
<CardHeader className="text-center border-0 pt-8 pt-md-4 pb-0 pb-md-4">
<div className="d-flex justify-content-between">
<Button
className="mr-4"
color="info"
href="javascript:;"
size="sm"
>
Connect
</Button>
<Button
className="float-right"
color="default"
href="javascript:;"
size="sm"
>
Message
</Button>
</div>
</CardHeader>
<CardBody className="pt-0">
<Row>
<div className="col">
<div className="card-profile-stats d-flex justify-content-center">
<div>
<span className="heading">22</span>
<span className="description">Friends</span>
</div>
<div>
<span className="heading">10</span>
<span className="description">Photos</span>
</div>
<div>
<span className="heading">89</span>
<span className="description">Comments</span>
</div>
</div>
</div>
</Row>
<div className="text-center">
<h5 className="h3">
Jessica Jones<span className="font-weight-light">, 27</span>
</h5>
<div className="h5 font-weight-300">
<i className="ni location_pin mr-2"></i>
Bucharest, Romania
</div>
</div>
</CardBody>
</Card>
</Col>
</Row>
</>
);
}
export default Example;
Contact PRO
import React from "react";
// reactstrap components
import { Button, Card, CardBody, Row, Col } from "reactstrap";
function Example() {
return (
<>
<Row>
<Col md="8">
<Card>
<CardBody>
<Row className="align-items-center">
<Col className="col-auto">
<a
className="avatar avatar-xl rounded-circle"
href="javascript:;"
>
<img
alt="..."
src={require("assets/img/theme/team-2.jpg")}
></img>
</a>
</Col>
<div className="col ml-2">
<h4 className="mb-0">
<a href="javascript:;">John Snow</a>
</h4>
<p className="text-sm text-muted mb-0">Working remoteley</p>
<span className="text-success">â</span>
<small>Active</small>
</div>
<Col className="col-auto">
<Button color="primary" size="sm" type="button">
Add
</Button>
</Col>
</Row>
</CardBody>
</Card>
</Col>
</Row>
</>
);
}
export default Example;
Team member PRO
import React from "react";
// reactstrap components
import { Button, Card, CardBody, Row, Col } from "reactstrap";
const imgStyle = { width: "140px" };
function Example() {
return (
<>
<Row>
<Col md="4">
<Card>
<CardBody>
<a href="javascript:;">
<img
alt="..."
className="rounded-circle img-center img-fluid shadow shadow-lg--hover"
src={require("assets/img/theme/team-1.jpg")}
style={imgStyle}
></img>
</a>
<div className="pt-4 text-center">
<h5 className="h3 title">
<span className="d-block mb-1">Ryan Tompson</span>
<small className="h4 font-weight-light text-muted">
Web Developer
</small>
</h5>
<div className="mt-3">
<Button
className="btn-icon-only rounded-circle"
color="twitter"
href="javascript:;"
>
<i className="fab fa-twitter"></i>
</Button>
<Button
className="btn-icon-only rounded-circle"
color="facebook"
href="javascript:;"
>
<i className="fab fa-facebook"></i>
</Button>
<Button
className="btn-icon-only rounded-circle"
color="dribbble"
href="javascript:;"
>
<i className="fab fa-dribbble"></i>
</Button>
</div>
</div>
</CardBody>
</Card>
</Col>
</Row>
</>
);
}
export default Example;
Image PRO
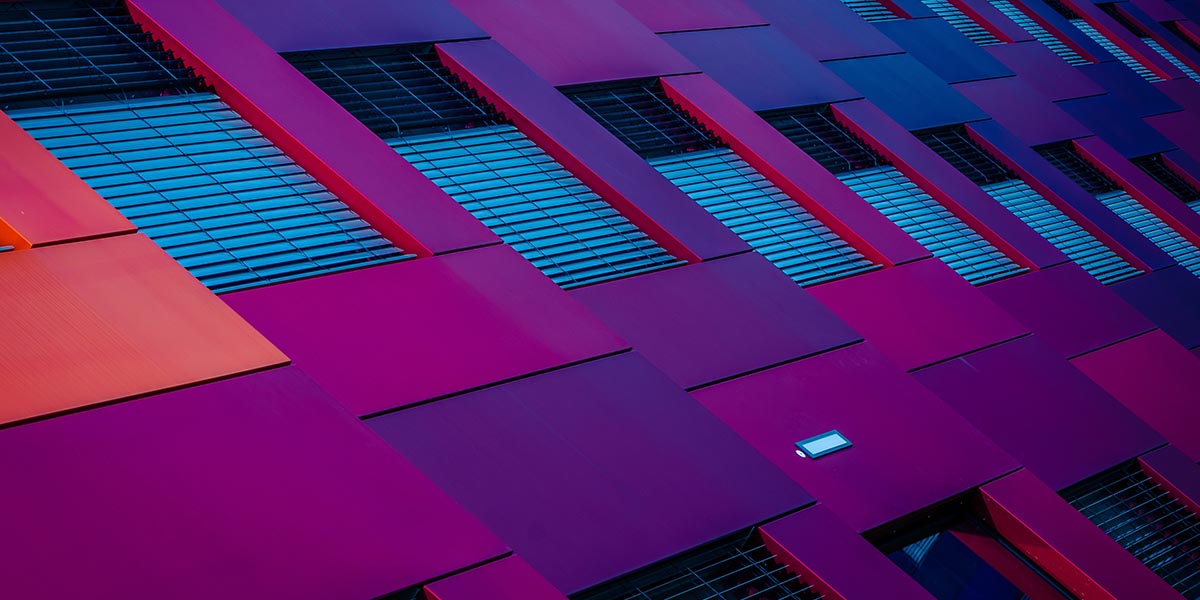
Get started with Argon
by John Snow on Oct 29th at 10:23 AMArgon is a great free UI package based on Bootstrap 4 that includes the most important components and features.
View articleimport React from "react";
// reactstrap components
import {
Button,
Card,
CardBody,
CardImg,
CardTitle,
CardText,
Row,
Col,
} from "reactstrap";
function Example() {
return (
<>
<Row>
<Col md="8">
<Card>
<CardImg
alt="..."
src={require("assets/img/theme/img-1-1000x600.jpg")}
top
></CardImg>
<CardBody>
<CardTitle className="h2 mb-0">Get started with Argon</CardTitle>
<small className="text-muted">
by John Snow on Oct 29th at 10:23 AM
</small>
<CardText className="mt-4">
Argon is a great free UI package based on Bootstrap 4 that
includes the most important components and features.
</CardText>
<Button className="px-0" color="link" href="javascript:;">
View article
</Button>
</CardBody>
</Card>
</Col>
</Row>
</>
);
}
export default Example;
Blockquote PRO
Testimonial
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Integer posuere erat a ante.
import React from "react";
// reactstrap components
import { Card, CardBody, CardTitle } from "reactstrap";
function Example() {
return (
<>
<Card className="bg-gradient-default">
<CardBody>
<CardTitle className="text-white" tag="h3">
Testimonial
</CardTitle>
<blockquote className="blockquote text-white mb-0">
<p>
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Integer
posuere erat a ante.
</p>
<footer className="blockquote-footer text-danger">
Someone famous in <cite title="Source Title">Source Title</cite>
</footer>
</blockquote>
</CardBody>
</Card>
</>
);
}
export default Example;
Pricing PRO
Bravo pack
-
Complete documentation
-
Working materials in Sketch
-
2GB cloud storage
import React from "react";
// reactstrap components
import {
Button,
Card,
CardHeader,
CardBody,
CardFooter,
Row,
Col,
} from "reactstrap";
function Example() {
return (
<>
<Row>
<Col md="6">
<Card className="card-pricing bg-gradient-success border-0 text-center mb-4">
<CardHeader className="bg-transparent">
<h4 className="text-uppercase ls-1 text-white py-3 mb-0">
Bravo pack
</h4>
</CardHeader>
<CardBody className="px-lg-7">
<div className="display-2 text-white">$49</div>
<span className="text-white">per application</span>
<ul className="list-unstyled my-4">
<li>
<div className="d-flex align-items-center">
<div>
<div className="icon icon-xs icon-shape bg-white shadow rounded-circle">
<i className="fas fa-terminal"></i>
</div>
</div>
<div>
<span className="pl-2 text-sm text-white">
Complete documentation
</span>
</div>
</div>
</li>
<li>
<div className="d-flex align-items-center">
<div>
<div className="icon icon-xs icon-shape bg-white shadow rounded-circle">
<i className="fas fa-pen-fancy"></i>
</div>
</div>
<div>
<span className="pl-2 text-sm text-white">
Working materials in Sketch
</span>
</div>
</div>
</li>
<li>
<div className="d-flex align-items-center">
<div>
<div className="icon icon-xs icon-shape bg-white shadow rounded-circle">
<i className="fas fa-hdd"></i>
</div>
</div>
<div>
<span className="pl-2 text-sm text-white">
2GB cloud storage
</span>
</div>
</div>
</li>
</ul>
<Button className="mb-3" color="primary" type="button">
Start free trial
</Button>
</CardBody>
<CardFooter className="bg-transparent">
<a className="text-white" href="javascript:;">
Request a demo
</a>
</CardFooter>
</Card>
</Col>
</Row>
</>
);
}
export default Example;
Overlay PRO
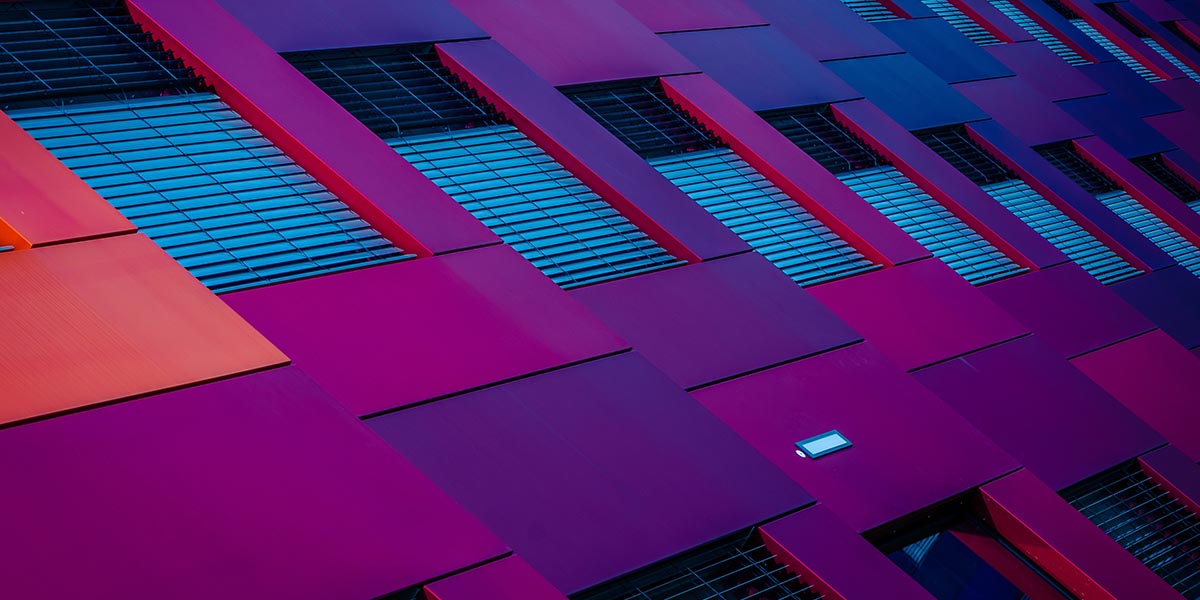
import React from "react";
// reactstrap components
import { Card, CardImg, CardImgOverlay, CardTitle, CardText } from "reactstrap";
function Example() {
return (
<>
<Card className="bg-dark text-white border-0">
<CardImg
alt="..."
src={require("assets/img/theme/img-1-1000x600.jpg")}
></CardImg>
<CardImgOverlay className="d-flex align-items-center">
<div>
<CardTitle className="h2 text-white mb-2">Card title</CardTitle>
<CardText>
This is a wider card with supporting text below as a natural
lead-in to additional content. This content is a little bit
longer.
</CardText>
<CardText className="text-sm font-weight-bold">
Last updated 3 mins ago
</CardText>
</div>
</CardImgOverlay>
</Card>
</>
);
}
export default Example;
Stats
Total traffic
350,8973.48% Since last month
New users
2,3563.48% Since last month
import React from "react";
// reactstrap components
import { Card, CardBody, CardTitle, Row, Col } from "reactstrap";
function Example() {
return (
<>
<Row className="mb-4">
<Col lg="6">
<Card className="card-stats">
<CardBody>
<Row>
<div className="col">
<CardTitle className="text-uppercase text-muted mb-0">
Total traffic
</CardTitle>
<span className="h2 font-weight-bold mb-0">350,897</span>
</div>
<Col className="col-auto">
<div className="icon icon-shape bg-red text-white rounded-circle shadow">
<i className="ni ni-active-40"></i>
</div>
</Col>
</Row>
<p className="mt-3 mb-0 text-sm">
<span className="text-success mr-2">
<i className="fa fa-arrow-up"></i>
3.48%
</span>
<span className="text-nowrap">Since last month</span>
</p>
</CardBody>
</Card>
</Col>
<Col lg="6">
<Card className="card-stats">
<CardBody>
<Row>
<div className="col">
<CardTitle className="text-uppercase text-muted mb-0">
New users
</CardTitle>
<span className="h2 font-weight-bold mb-0">2,356</span>
</div>
<Col className="col-auto">
<div className="icon icon-shape bg-orange text-white rounded-circle shadow">
<i className="ni ni-chart-pie-35"></i>
</div>
</Col>
</Row>
<p className="mt-3 mb-0 text-sm">
<span className="text-success mr-2">
<i className="fa fa-arrow-up"></i>
3.48%
</span>
<span className="text-nowrap">Since last month</span>
</p>
</CardBody>
</Card>
</Col>
</Row>
</>
);
}
export default Example;
Total traffic
350,8973.48% Since last month
New users
2,3563.48% Since last month
import React from "react";
// reactstrap components
import { Card, CardBody, CardTitle, Row, Col } from "reactstrap";
function Example() {
return (
<>
<Row className="mb-4">
<Col lg="6">
<Card className="bg-gradient-default">
<CardBody>
<Row>
<div className="col">
<CardTitle className="text-uppercase text-muted mb-0 text-white">
Total traffic
</CardTitle>
<span className="h2 font-weight-bold mb-0 text-white">
350,897
</span>
</div>
<Col className="col-auto">
<div className="icon icon-shape bg-white text-dark rounded-circle shadow">
<i className="ni ni-active-40"></i>
</div>
</Col>
</Row>
<p className="mt-3 mb-0 text-sm">
<span className="text-white mr-2">
<i className="fa fa-arrow-up"></i>
3.48%
</span>
<span className="text-nowrap text-light">
Since last month
</span>
</p>
</CardBody>
</Card>
</Col>
<Col lg="6">
<Card className="bg-gradient-primary">
<CardBody>
<Row>
<div className="col">
<CardTitle className="text-uppercase text-muted mb-0 text-white">
New users
</CardTitle>
<span className="h2 font-weight-bold mb-0 text-white">
2,356
</span>
</div>
<Col className="col-auto">
<div className="icon icon-shape bg-white text-dark rounded-circle shadow">
<i className="ni ni-atom"></i>
</div>
</Col>
</Row>
<p className="mt-3 mb-0 text-sm">
<span className="text-white mr-2">
<i className="fa fa-arrow-up"></i>
3.48%
</span>
<span className="text-nowrap text-light">
Since last month
</span>
</p>
</CardBody>
</Card>
</Col>
</Row>
</>
);
}
export default Example;
Background Image
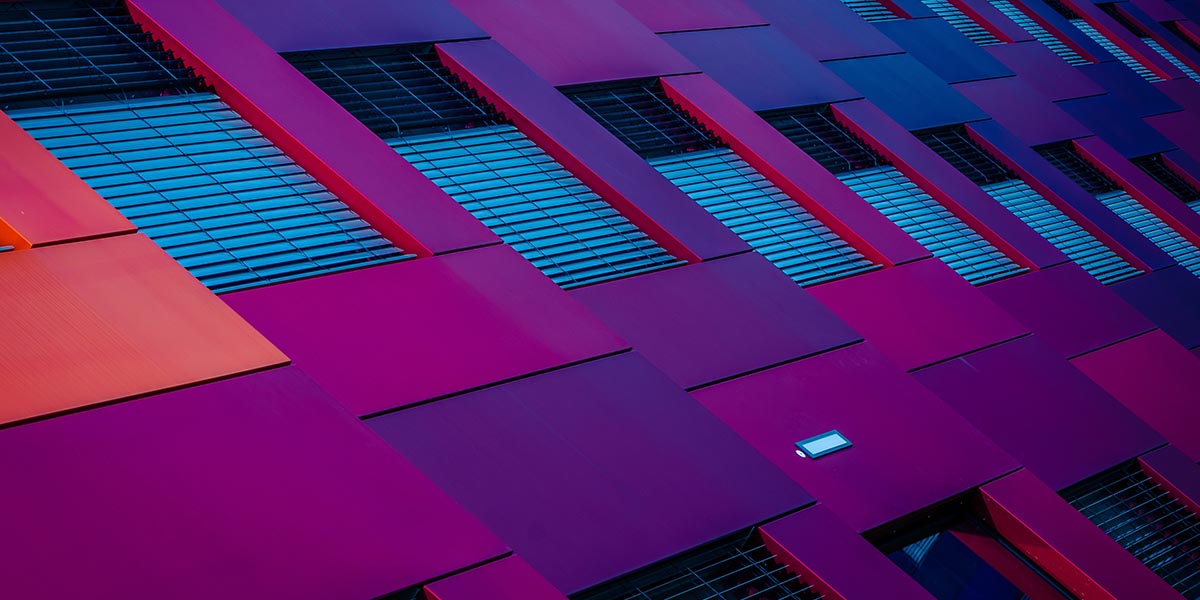
import React from "react";
// reactstrap components
import { Card, CardImg } from "reactstrap";
function Example() {
return (
<>
<Card className="bg-dark text-white border-0">
<CardImg
alt="..."
src={require("assets/img/theme/img-1-1000x600.jpg")}
></CardImg>
</Card>
</>
);
}
export default Example;
Props
If you want to see more examples and properties please check the official Reactstrap Documentation.