Nextjs List Group
-List groups are a flexible and powerful component for displaying a series of content. With Nextjs list group you can modify and extend them to support just about any content within.
Examples
The most basic list group is an unordered list with list items and the proper classes. Build upon it with the options that follow, or with your own CSS as needed.
- Cras justo odio
- Dapibus ac facilisis in
- Morbi leo risus
- Porta ac consectetur ac
- Vestibulum at eros
import React from "react";
// reactstrap components
import { ListGroupItem, ListGroup } from "reactstrap";
function Example() {
return (
<>
<ListGroup>
<ListGroupItem>Cras justo odio</ListGroupItem>
<ListGroupItem>Dapibus ac facilisis in</ListGroupItem>
<ListGroupItem>Morbi leo risus</ListGroupItem>
<ListGroupItem>Porta ac consectetur ac</ListGroupItem>
<ListGroupItem>Vestibulum at eros</ListGroupItem>
</ListGroup>
</>
);
}
export default Example;
Active items
Add
.active
to
a
.list-group-item
to indicate the current active selection.
- Cras justo odio
- Dapibus ac facilisis in
- Morbi leo risus
- Porta ac consectetur ac
- Vestibulum at eros
import React from "react";
// reactstrap components
import { ListGroupItem, ListGroup } from "reactstrap";
function Example() {
return (
<>
<ListGroup>
<ListGroupItem className=" active">Cras justo odio</ListGroupItem>
<ListGroupItem>Dapibus ac facilisis in</ListGroupItem>
<ListGroupItem>Morbi leo risus</ListGroupItem>
<ListGroupItem>Porta ac consectetur ac</ListGroupItem>
<ListGroupItem>Vestibulum at eros</ListGroupItem>
</ListGroup>
</>
);
}
export default Example;
Links
import React from "react";
// reactstrap components
import { ListGroupItem, ListGroup } from "reactstrap";
function Example() {
return (
<>
<ListGroup>
<ListGroupItem
className=" list-group-item-action active"
href="#pablo"
onClick={(e) => e.preventDefault()}
tag="a"
>
Cras justo odio
</ListGroupItem>
<ListGroupItem
className=" list-group-item-action"
href="#pablo"
onClick={(e) => e.preventDefault()}
tag="a"
>
Dapibus ac facilisis in
</ListGroupItem>
<ListGroupItem
className=" list-group-item-action"
href="#pablo"
onClick={(e) => e.preventDefault()}
tag="a"
>
Morbi leo risus
</ListGroupItem>
<ListGroupItem
className=" list-group-item-action"
href="#pablo"
onClick={(e) => e.preventDefault()}
tag="a"
>
Porta ac consectetur ac
</ListGroupItem>
<ListGroupItem
className=" list-group-item-action disabled"
href="#pablo"
onClick={(e) => e.preventDefault()}
tag="a"
>
Vestibulum at eros
</ListGroupItem>
</ListGroup>
</>
);
}
export default Example;
With badges
- Cras justo odio 14
- Dapibus ac facilisis in 2
- Morbi leo risus 1
import React from "react";
// reactstrap components
import { Badge, ListGroupItem, ListGroup } from "reactstrap";
function Example() {
return (
<>
<ListGroup>
<ListGroupItem className=" d-flex justify-content-between align-items-center">
Cras justo odio{" "}
<Badge color="primary" pill>
14
</Badge>
</ListGroupItem>
<ListGroupItem className=" d-flex justify-content-between align-items-center">
Dapibus ac facilisis in{" "}
<Badge color="primary" pill>
2
</Badge>
</ListGroupItem>
<ListGroupItem className=" d-flex justify-content-between align-items-center">
Morbi leo risus{" "}
<Badge color="primary" pill>
1
</Badge>
</ListGroupItem>
</ListGroup>
</>
);
}
export default Example;
Advanced Examples
Here are some more advanced custom examples we’ve made in order to bring more functionality with some really cool list group examples.
Members
import React from "react";
// reactstrap components
import { Button, ListGroupItem, ListGroup, Row, Col } from "reactstrap";
function Example() {
return (
<>
<ListGroup className=" list my--3" flush>
<ListGroupItem className=" px-0">
<Row className=" align-items-center">
<Col className=" col-auto">
<a className=" avatar rounded-circle" href="javascript:;">
<img
alt="..."
src={require("assets/img/theme/team-1.jpg")}
></img>
</a>
</Col>
<div className=" col ml--2">
<h4 className=" mb-0">
<a href="javascript:;">John Michael</a>
</h4>
<span className=" text-success">â</span>
<small>Online</small>
</div>
<Col className=" col-auto">
<Button color="primary" size="sm" type="button">
Add
</Button>
</Col>
</Row>
</ListGroupItem>
<ListGroupItem className=" px-0">
<Row className=" align-items-center">
<Col className=" col-auto">
<a className=" avatar rounded-circle" href="javascript:;">
<img
alt="..."
src={require("assets/img/theme/team-2.jpg")}
></img>
</a>
</Col>
<div className=" col ml--2">
<h4 className=" mb-0">
<a href="javascript:;">Alex Smith</a>
</h4>
<span className=" text-warning">â</span>
<small>In a meeting</small>
</div>
<Col className=" col-auto">
<Button color="primary" size="sm" type="button">
Add
</Button>
</Col>
</Row>
</ListGroupItem>
<ListGroupItem className=" px-0">
<Row className=" align-items-center">
<Col className=" col-auto">
<a className=" avatar rounded-circle" href="javascript:;">
<img
alt="..."
src={require("assets/img/theme/team-3.jpg")}
></img>
</a>
</Col>
<div className=" col ml--2">
<h4 className=" mb-0">
<a href="javascript:;">Samantha Ivy</a>
</h4>
<span className=" text-danger">â</span>
<small>Offline</small>
</div>
<Col className=" col-auto">
<Button color="primary" size="sm" type="button">
Add
</Button>
</Col>
</Row>
</ListGroupItem>
<ListGroupItem className=" px-0">
<Row className=" align-items-center">
<Col className=" col-auto">
<a className=" avatar rounded-circle" href="javascript:;">
<img
alt="..."
src={require("assets/img/theme/team-4.jpg")}
></img>
</a>
</Col>
<div className=" col ml--2">
<h4 className=" mb-0">
<a href="javascript:;">John Michael</a>
</h4>
<span className=" text-success">â</span>
<small>Online</small>
</div>
<Col className=" col-auto">
<Button color="primary" size="sm" type="button">
Add
</Button>
</Col>
</Row>
</ListGroupItem>
<ListGroupItem className=" px-0">
<Row className=" align-items-center">
<Col className=" col-auto">
<a className=" avatar rounded-circle" href="javascript:;">
<img
alt="..."
src={require("assets/img/theme/team-5.jpg")}
></img>
</a>
</Col>
<div className=" col ml--2">
<h4 className=" mb-0">
<a href="javascript:;">John Snow</a>
</h4>
<span className=" text-success">â</span>
<small>Online</small>
</div>
<Col className=" col-auto">
<Button color="primary" size="sm" type="button">
Add
</Button>
</Col>
</Row>
</ListGroupItem>
</ListGroup>
</>
);
}
export default Example;
Checklist
-
Call with Dave
10:30 AM -
Lunch meeting
10:30 AM -
Argon Dashboard Launch
10:30 AM -
Winter Hackaton
10:30 AM -
Dinner with Family
10:30 AM
import React from "react";
// reactstrap components
import { ListGroupItem, ListGroup } from "reactstrap";
function Example() {
return (
<>
<ListGroup data-toggle="checklist" flush>
<ListGroupItem className=" checklist-entry flex-column align-items-start py-4 px-4">
<div className=" checklist-item checklist-item-success">
<div className=" checklist-info">
<h5 className=" checklist-title mb-0">Call with Dave</h5>
<small>10:30 AM</small>
</div>
<div>
<div className=" custom-control custom-checkbox custom-checkbox-success">
<input
className=" custom-control-input"
defaultChecked
id="chk-todo-task-1"
type="checkbox"
></input>
<label
className=" custom-control-label"
htmlFor="chk-todo-task-1"
></label>
</div>
</div>
</div>
</ListGroupItem>
<ListGroupItem className=" checklist-entry flex-column align-items-start py-4 px-4">
<div className=" checklist-item checklist-item-warning">
<div className=" checklist-info">
<h5 className=" checklist-title mb-0">Lunch meeting</h5>
<small>10:30 AM</small>
</div>
<div>
<div className=" custom-control custom-checkbox custom-checkbox-warning">
<input
className=" custom-control-input"
id="chk-todo-task-2"
type="checkbox"
></input>
<label
className=" custom-control-label"
htmlFor="chk-todo-task-2"
></label>
</div>
</div>
</div>
</ListGroupItem>
<ListGroupItem className=" checklist-entry flex-column align-items-start py-4 px-4">
<div className=" checklist-item checklist-item-info">
<div className=" checklist-info">
<h5 className=" checklist-title mb-0">Argon Dashboard Launch</h5>
<small>10:30 AM</small>
</div>
<div>
<div className=" custom-control custom-checkbox custom-checkbox-info">
<input
className=" custom-control-input"
id="chk-todo-task-3"
type="checkbox"
></input>
<label
className=" custom-control-label"
htmlFor="chk-todo-task-3"
></label>
</div>
</div>
</div>
</ListGroupItem>
<ListGroupItem className=" checklist-entry flex-column align-items-start py-4 px-4">
<div className=" checklist-item checklist-item-danger">
<div className=" checklist-info">
<h5 className=" checklist-title mb-0">Winter Hackaton</h5>
<small>10:30 AM</small>
</div>
<div>
<div className=" custom-control custom-checkbox custom-checkbox-danger">
<input
className=" custom-control-input"
defaultChecked
id="chk-todo-task-4"
type="checkbox"
></input>
<label
className=" custom-control-label"
htmlFor="chk-todo-task-4"
></label>
</div>
</div>
</div>
</ListGroupItem>
<ListGroupItem className=" checklist-entry flex-column align-items-start py-4 px-4">
<div className=" checklist-item checklist-item-success">
<div className=" checklist-info">
<h5 className=" checklist-title mb-0">Dinner with Family</h5>
<small>10:30 AM</small>
</div>
<div>
<div className=" custom-control custom-checkbox custom-checkbox-success">
<input
className=" custom-control-input"
defaultChecked
id="chk-todo-task-5"
type="checkbox"
></input>
<label
className=" custom-control-label"
htmlFor="chk-todo-task-5"
></label>
</div>
</div>
</div>
</ListGroupItem>
</ListGroup>
</>
);
}
export default Example;
Progress
import React from "react";
// reactstrap components
import { ListGroupItem, ListGroup, Progress, Row, Col } from "reactstrap";
function Example() {
return (
<>
<ListGroup className=" list my-3" flush>
<ListGroupItem className=" px-0">
<Row className=" align-items-center">
<Col className=" col-auto">
<a className=" avatar rounded-circle" href="javascript:;">
<img
alt="..."
src={require("assets/img/theme/bootstrap.jpg")}
></img>
</a>
</Col>
<div className=" col">
<h5>Argon Design System</h5>
<Progress
barClassName=" progress-xs mb-0"
max="100"
value="60"
></Progress>
</div>
</Row>
</ListGroupItem>
<ListGroupItem className=" px-0">
<Row className=" align-items-center">
<Col className=" col-auto">
<a className=" avatar rounded-circle" href="javascript:;">
<img
alt="..."
src={require("assets/img/theme/angular.jpg")}
></img>
</a>
</Col>
<div className=" col">
<h5>Angular Now UI Kit PRO</h5>
<Progress
barClassName=" progress-xs mb-0"
max="100"
value="100"
></Progress>
</div>
</Row>
</ListGroupItem>
<ListGroupItem className=" px-0">
<Row className=" align-items-center">
<Col className=" col-auto">
<a className=" avatar rounded-circle" href="javascript:;">
<img
alt="..."
src={require("assets/img/theme/sketch.jpg")}
></img>
</a>
</Col>
<div className=" col">
<h5>Black Dashboard</h5>
<Progress
barClassName=" progress-xs mb-0"
max="100"
value="72"
></Progress>
</div>
</Row>
</ListGroupItem>
<ListGroupItem className=" px-0">
<Row className=" align-items-center">
<Col className=" col-auto">
<a className=" avatar rounded-circle" href="javascript:;">
<img
alt="..."
src={require("assets/img/theme/react.jpg")}
></img>
</a>
</Col>
<div className=" col">
<h5>React Material Dashboard</h5>
<Progress
barClassName=" progress-xs mb-0"
max="100"
value="90"
></Progress>
</div>
</Row>
</ListGroupItem>
<ListGroupItem className=" px-0">
<Row className=" align-items-center">
<Col className=" col-auto">
<a className=" avatar rounded-circle" href="javascript:;">
<img alt="..." src={require("assets/img/theme/vue.jpg")}></img>
</a>
</Col>
<div className=" col">
<h5>Vue Paper UI Kit PRO</h5>
<Progress
barClassName=" progress-xs mb-0"
max="100"
value="100"
></Progress>
</div>
</Row>
</ListGroupItem>
</ListGroup>
</>
);
}
export default Example;
Messages
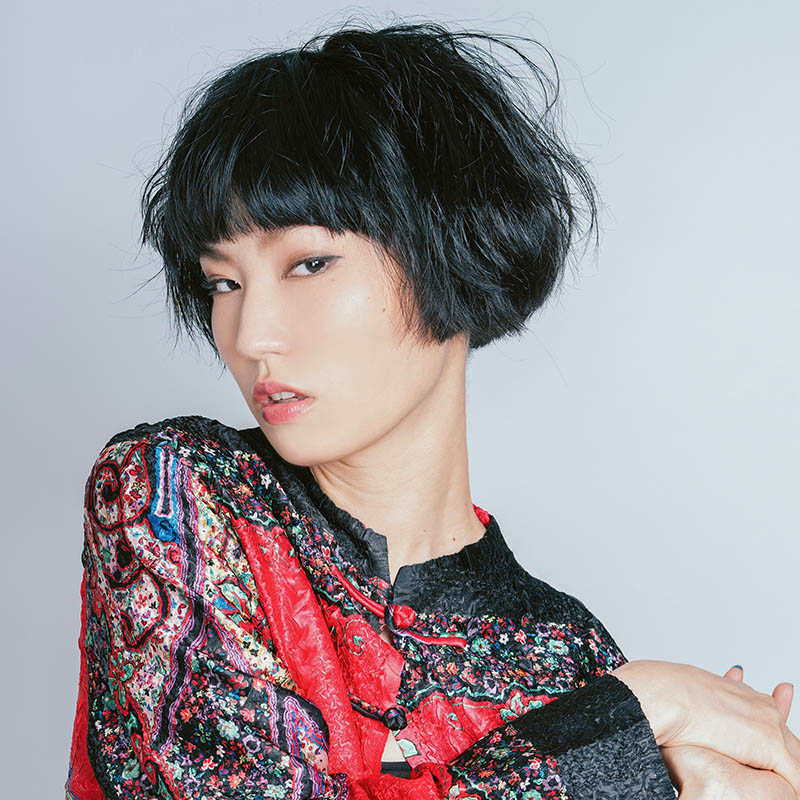
Tim
New order for Argon Dashboard
Doasdnec id elit non mi porta gravida at eget metus. Maecenas sed diam eget risus varius blandit.
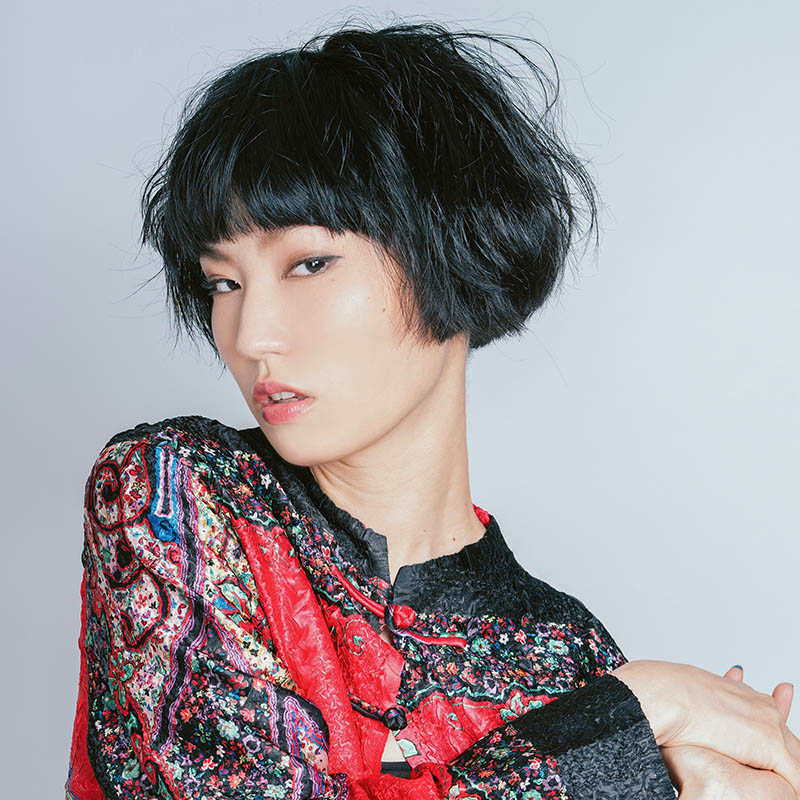
Mike
● Your theme has been updated
Doasdnec id elit non mi porta gravida at eget metus. Maecenas sed diam eget risus varius blandit.
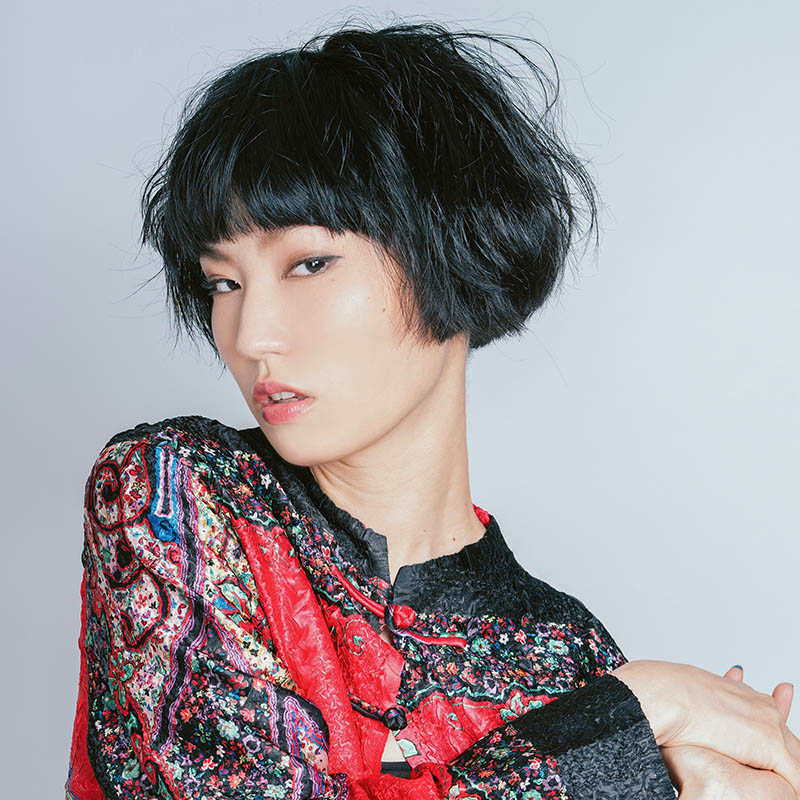
Tim
New order for Argon Dashboard
Doasdnec id elit non mi porta gravida at eget metus. Maecenas sed diam eget risus varius blandit.
import React from "react";
// reactstrap components
import { ListGroupItem, ListGroup } from "reactstrap";
function Example() {
return (
<>
<ListGroup flush>
<ListGroupItem
className=" list-group-item-action flex-column align-items-start py-4 px-4"
href="javascript:;"
tag="a"
>
<div className=" d-flex w-100 justify-content-between">
<div>
<div className=" d-flex w-100 align-items-center">
<img
alt="..."
className=" avatar avatar-xs mr-2"
src={require("assets/img/theme/team-3.jpg")}
></img>
<h5 className=" mb-1">Tim</h5>
</div>
</div>
<small>2 hrs ago</small>
</div>
<h4 className=" mt-3 mb-1">New order for Argon Dashboard</h4>
<p className=" text-sm mb-0">
Doasdnec id elit non mi porta gravida at eget metus. Maecenas sed
diam eget risus varius blandit.
</p>
</ListGroupItem>
<ListGroupItem
className=" list-group-item-action flex-column align-items-start py-4 px-4"
href="javascript:;"
tag="a"
>
<div className=" d-flex w-100 justify-content-between">
<div>
<div className=" d-flex w-100 align-items-center">
<img
alt="..."
className=" avatar avatar-xs mr-2"
src={require("assets/img/theme/team-3.jpg")}
></img>
<h5 className=" mb-1">Mike</h5>
</div>
</div>
<small>1 day ago</small>
</div>
<h4 className=" mt-3 mb-1">
<span className=" text-info">â</span>
Your theme has been updated
</h4>
<p className=" text-sm mb-0">
Doasdnec id elit non mi porta gravida at eget metus. Maecenas sed
diam eget risus varius blandit.
</p>
</ListGroupItem>
<ListGroupItem
className=" list-group-item-action flex-column align-items-start py-4 px-4"
href="javascript:;"
tag="a"
>
<div className=" d-flex w-100 justify-content-between">
<div>
<div className=" d-flex w-100 align-items-center">
<img
alt="..."
className=" avatar avatar-xs mr-2"
src={require("assets/img/theme/team-3.jpg")}
></img>
<h5 className=" mb-1">Tim</h5>
</div>
</div>
<small>2 hrs ago</small>
</div>
<h4 className=" mt-3 mb-1">New order for Argon Dashboard</h4>
<p className=" text-sm mb-0">
Doasdnec id elit non mi porta gravida at eget metus. Maecenas sed
diam eget risus varius blandit.
</p>
</ListGroupItem>
</ListGroup>
</>
);
}
export default Example;
Notifications
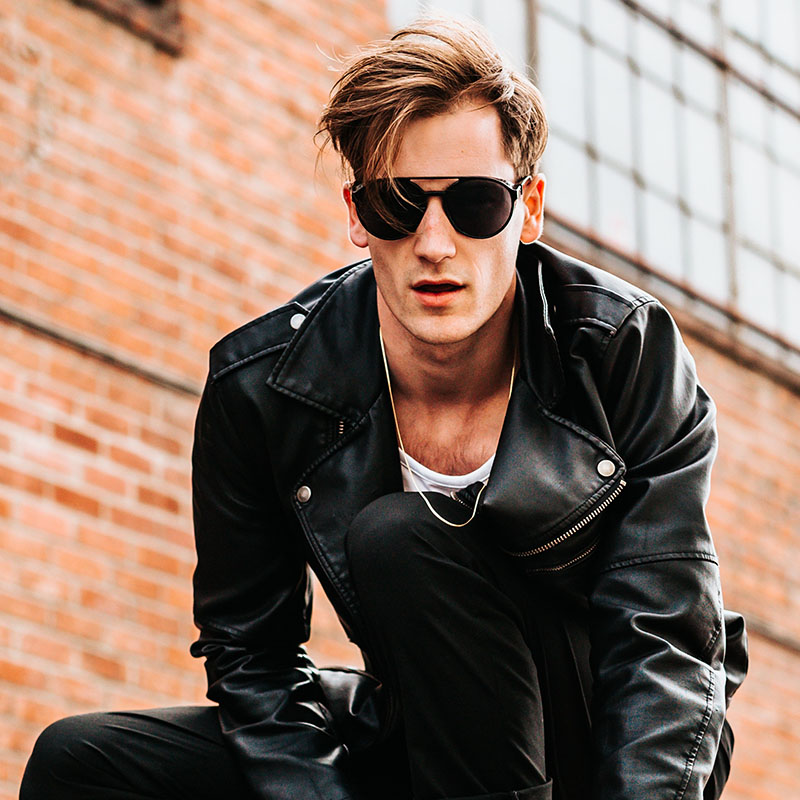
John Snow
Let's meet at Starbucks at 11:30. Wdyt?
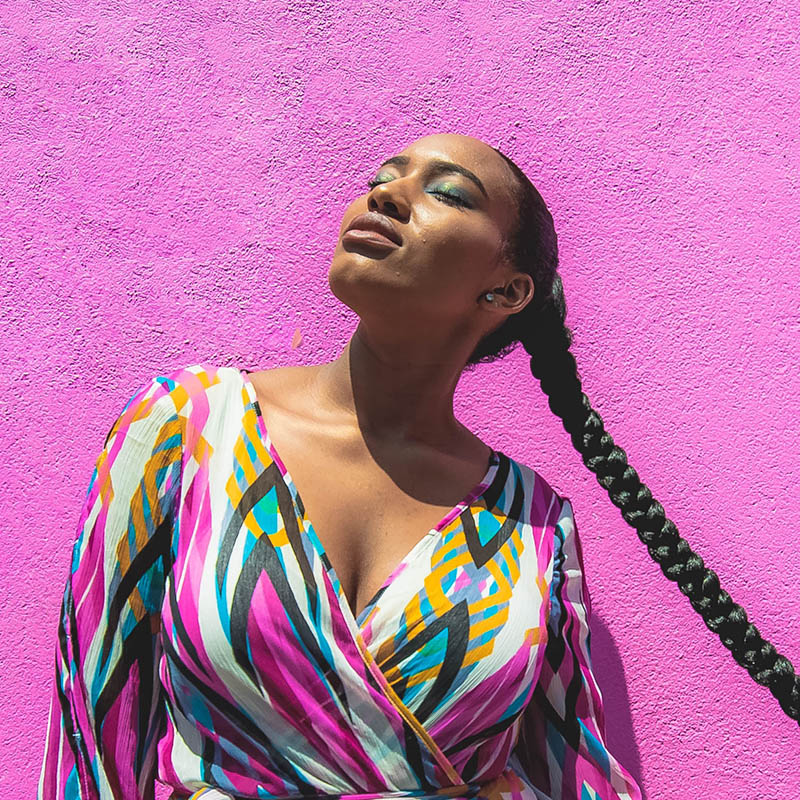
John Snow
A new issue has been reported for Argon.
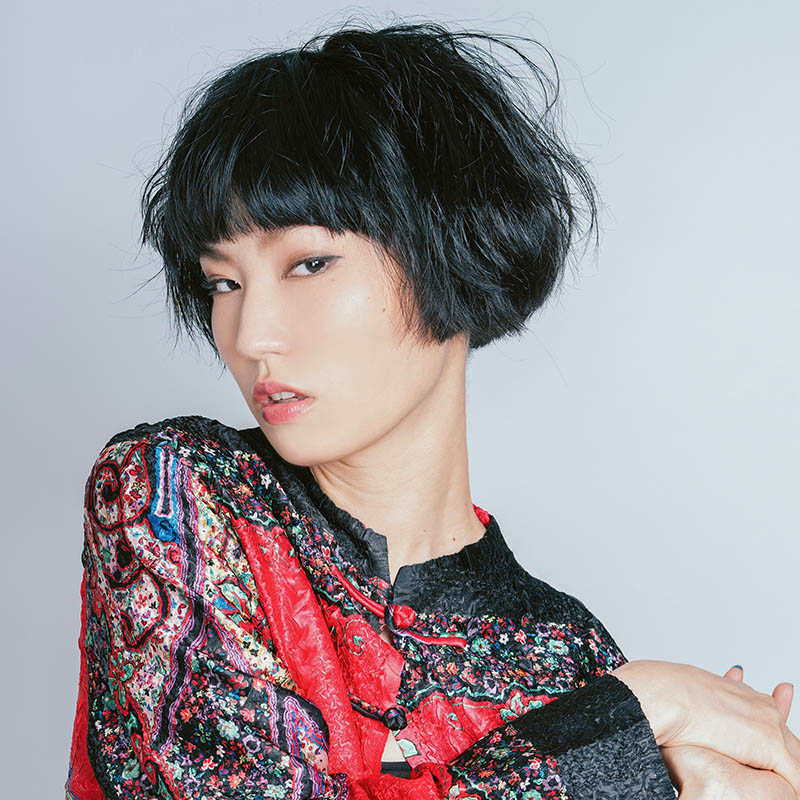
John Snow
Your posts have been liked a lot.
import React from "react";
// reactstrap components
import { ListGroupItem, ListGroup, Row, Col } from "reactstrap";
function Example() {
return (
<>
<ListGroup flush>
<ListGroupItem
className=" list-group-item-action"
href="javascript:;"
tag="a"
>
<Row className=" align-items-center">
<Col className=" col-auto">
<img
alt="..."
className=" avatar rounded-circle"
src={require("assets/img/theme/team-1.jpg")}
></img>
</Col>
<div className=" col ml--2">
<div className=" d-flex justify-content-between align-items-center">
<div>
<h4 className=" mb-0 text-sm">John Snow</h4>
</div>
<div className=" text-right text-muted">
<small>2 hrs ago</small>
</div>
</div>
<p className=" text-sm mb-0">
Let's meet at Starbucks at 11:30. Wdyt?
</p>
</div>
</Row>
</ListGroupItem>
<ListGroupItem
className=" list-group-item-action"
href="javascript:;"
tag="a"
>
<Row className=" align-items-center">
<Col className=" col-auto">
<img
alt="..."
className=" avatar rounded-circle"
src={require("assets/img/theme/team-2.jpg")}
></img>
</Col>
<div className=" col ml--2">
<div className=" d-flex justify-content-between align-items-center">
<div>
<h4 className=" mb-0 text-sm">John Snow</h4>
</div>
<div className=" text-right text-muted">
<small>3 hrs ago</small>
</div>
</div>
<p className=" text-sm mb-0">
A new issue has been reported for Argon.
</p>
</div>
</Row>
</ListGroupItem>
<ListGroupItem
className=" list-group-item-action"
href="javascript:;"
tag="a"
>
<Row className=" align-items-center">
<Col className=" col-auto">
<img
alt="..."
className=" avatar rounded-circle"
src={require("assets/img/theme/team-3.jpg")}
></img>
</Col>
<div className=" col ml--2">
<div className=" d-flex justify-content-between align-items-center">
<div>
<h4 className=" mb-0 text-sm">John Snow</h4>
</div>
<div className=" text-right text-muted">
<small>5 hrs ago</small>
</div>
</div>
<p className=" text-sm mb-0">Your posts have been liked a lot.</p>
</div>
</Row>
</ListGroupItem>
</ListGroup>
</>
);
}
export default Example;
Countries
-
Country:
United States
Visits:2500
Bounce:30%
-
Country:
Germany
Visits:2500
Bounce:30%
-
Country:
Great Britain
Visits:2500
Bounce:30%
import React from "react";
// reactstrap components
import { ListGroupItem, ListGroup, Row, Col } from "reactstrap";
function Example() {
return (
<>
<ListGroup className=" list my--3" flush>
<ListGroupItem className=" px-0">
<Row className=" align-items-center">
<Col className=" col-auto">
<img
alt="..."
src={require("assets/img/icons/flags/US.png")}
></img>
</Col>
<div className=" col">
<small>Country:</small>
<h5 className=" mb-0">United States</h5>
</div>
<div className=" col">
<small>Visits:</small>
<h5 className=" mb-0">2500</h5>
</div>
<div className=" col">
<small>Bounce:</small>
<h5 className=" mb-0">30%</h5>
</div>
</Row>
</ListGroupItem>
<ListGroupItem className=" px-0">
<Row className=" align-items-center">
<Col className=" col-auto">
<img
alt="..."
src={require("assets/img/icons/flags/DE.png")}
></img>
</Col>
<div className=" col">
<small>Country:</small>
<h5 className=" mb-0">Germany</h5>
</div>
<div className=" col">
<small>Visits:</small>
<h5 className=" mb-0">2500</h5>
</div>
<div className=" col">
<small>Bounce:</small>
<h5 className=" mb-0">30%</h5>
</div>
</Row>
</ListGroupItem>
<ListGroupItem className=" px-0">
<Row className=" align-items-center">
<Col className=" col-auto">
<img
alt="..."
src={require("assets/img/icons/flags/GB.png")}
></img>
</Col>
<div className=" col">
<small>Country:</small>
<h5 className=" mb-0">Great Britain</h5>
</div>
<div className=" col">
<small>Visits:</small>
<h5 className=" mb-0">2500</h5>
</div>
<div className=" col">
<small>Bounce:</small>
<h5 className=" mb-0">30%</h5>
</div>
</Row>
</ListGroupItem>
</ListGroup>
</>
);
}
export default Example;
Props
If you want to see more examples and properties please check the official Reactstrap Documentation.