From completing tasks for you to setting up automated responses similar to that of an IVR system, a great virtual assistant can make your life much easier.
The good news is that it’s easy to create your own with a bit of coding. Building your dream virtual assistant using Python can become a reality as Python offers a robust library for creating a virtual assistant that works for you.
What do you want your virtual assistant to do?
First of all, consider what tasks you’d like your virtual assistant to complete. This may include:
- Weather forecasting
- Launching website applications
- Generating personalized email responses
- Executing commands with voice prompts
- Reading emails using text-to-speech technology
- Searching for and playing music
The possibilities are pretty much endless.
Building your virtual assistant using Python
Before you begin, you’ll need to install a recent version of Python. A typical use case is ActivePython, and you can either:
- Download and install the pre-built Virtual Assistant runtime environment, or;
- Build a custom Python runtime by creating a free ActiveState Platform account.
Once you have installed Python, click the Get Started button and select Python 3.6 and your OS.
As well as ActivePython, you’ll need to include a few third-party packages that allow for speech recognition, playback audio, and converting text to speech. A speech recognition package allows Python to access audio from your microphone, transcribe, and save audio. An audio playback package enables Python to play back your MP3 files. Meanwhile, a text-to-speech package converts your voiced question to text and then converts the response into a voiceable phrase.
Once you’ve followed these steps to install Python correctly, you can start to build the sample application.
Voice input
The first step to creating your own virtual assistant using Python is establishing voice communication. Within the installed libraries, you’ll need two functions – one for listening and another for responding.
To do this, you’ll need to import the libraries, together with a few of the standard Python libraries.
import speech_recognition as sr
from time import ctime
import time
import os
from gtts import gTTS
import requests, json
The next step is to define the listening function:
def listen():
r = sr.Recognizer()
with sr.Microphone() as source:
print("I am listening...")
audio = r.listen(source)
data = ""
try:
data = r.recognize_google(audio)
print("You said: " + data)
except sr.UnknownValueError:
print("Google Speech Recognition did not understand audio")
except sr.RequestError as e:
print("Request Failed; {0}".format(e))
return data
Next, define the voice response as follows:
def respond(audioString):
print(audioString)
tts = gTTS(text=audioString, lang='en')
tts.save("speech.mp3")
os.system("mpg321 speech.mp3")
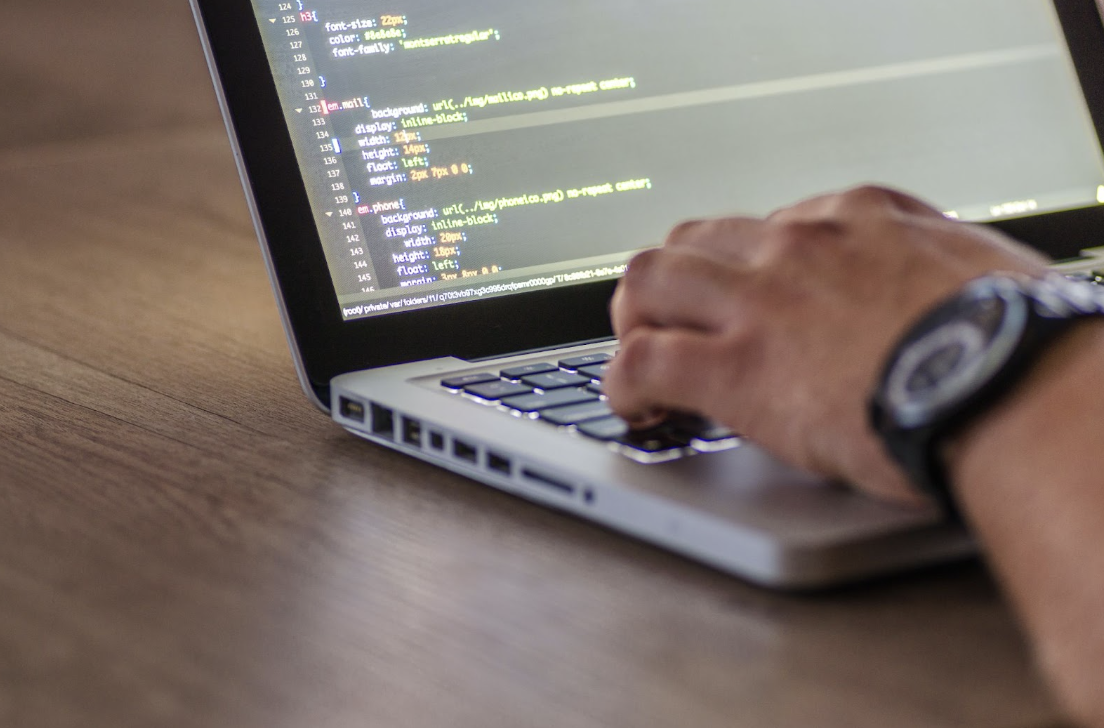
Now that these functions are defined, we can move on to building your virtual assistant as an efficient web tool, with a few additional features. Let’s start with voiced responses:
def digital_assistant(data):
if "how are you" in data:
listening = True
respond("I am well")
if "what time is it" in data:
listening = True
respond(ctime())
if "stop listening" in data:
listening = False
print('Listening stopped')
return listening
return listening
This feature uses the listen function outputs as an input to verify what was said. You can use a series of “if” statements to understand the voice query and output the most relevant response. For example, to make your virtual assistant come to life, you can add an automated response to the question “How are you doing?”.
This is how it looks:
while True:
res = obj.mic_input()
if re.search('hello', res):
print('Hi')
t2s('Hi')
if re.search('how are you', res):
li = ['ok', 'fine', 'wonderful']
response = random.choice(li)
print(f"I am {response}")
t2s(f"I am {response}")
if re.search('your name|who are you', res):
print("My name is Hannah, I am your personal assistant")
t2s("My name is Hannah, I am your personal assistant")
If you are a backend developer conversant with Python, this will be a simple task for you.
Open a website
Next up, let’s look at how your virtual assistant can open website applications for you.
‘obj.website_opener(domain)’ will open any website. To create this function, fetch the domain from user input, then pass to ‘obj.website_opener(domain)’ to open in your default browser.
Get the date
Another useful function for your dream virtual assistant is for it to retrieve the date for you upon request. In this example, we’ll create a function to return today’s date as a string:
def getDate():
now = datetime.datetime.now()
my_date = datetime.datetime.today()
weekday = calendar.day_name[my_date.weekday()]# e.g. Monday
monthNum = now.month
dayNum = now.day
month_names = ['January', 'February', 'March', 'April', 'May',
'June', 'July', 'August', 'September', 'October', 'November',
'December']
ordinalNumbers = ['1st', '2nd', '3rd', '4th', '5th', '6th',
'7th', '8th', '9th', '10th', '11th', '12th',
'13th', '14th', '15th', '16th', '17th',
'18th', '19th', '20th', '21st', '22nd',
'23rd', '24th', '25th', '26th', '27th',
'28th', '29th', '30th', '31st']
return 'Today is ' + weekday + ' ' + month_names[monthNum - 1] + ' the ' + ordinalNumbers[dayNum - 1] + '.'
Fetch date from Wikipedia
The following command enables your virtual assistant to fetch data from Wikipedia. The wikipedia.summary() function takes two arguments – the statement given by the user, and the number of sentences from Wikipedia that are required to be stored as a variable result:
if 'wikipedia' in statement:
speak('Searching Wikipedia...')
statement =statement.replace("wikipedia", "")
results = wikipedia.summary(statement, sentences=3)
speak("According to Wikipedia")
print(results)
speak(results)
Some developers create functions around typical customer touchpoints for the most common and appropriate responses. However, there are several opportunities to add more functionalities to your virtual assistant and customize it to your exact requirements.
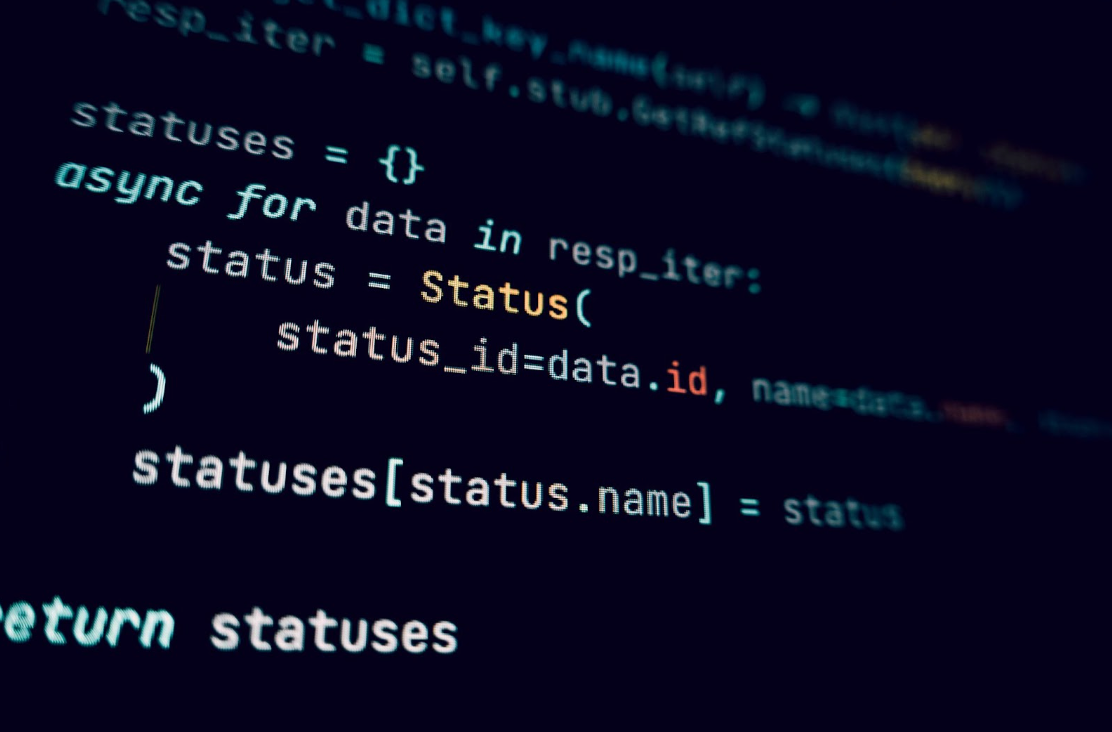
Log off your PC
Use the subprocess.call() function to process the system function to log off or turn off your computer. This command tells your virtual assistant to automatically turn off your PC:
elif "log off" in statement or "sign out" in statement:
speak("Ok , your pc will log off in 10 sec make sure you exit from all applications")
subprocess.call(["shutdown", "/l"])
time.sleep(3)
Your dream virtual assistant awaits!
A dream virtual assistant is similar to typical customer service representative duties which include resolving customers’ questions quickly and effectively. Build your virtual assistant using Python, and you’ll have a perfect companion to answer your every query. Good luck!
Author Bio
Jessica Day is the Senior Director for Marketing Strategy at Dialpad, a call routing service provider and a modern business communications platform that takes every kind of conversation to the next level—turning conversations into opportunities. Jessica is an expert in collaborating with multifunctional teams to execute and optimize marketing efforts for both company and client campaigns. Jessica has written for domains such as DSers and Training Industry. Here is her LinkedIn.