This article is just another Django Cheat Sheet that might help beginners to speed up their learning curve and code much faster projects with commercial value. For newcomers, Django is the most popular Python-based web framework initially released in 2003. Since then, Django has become a reference framework in the web development ecosystem mostly for the “batteries-included” concept and built-in security patterns coded by experienced developers. To make this article more useful and trigger curious minds into programming, a few open-source Django projects will be mentioned.
Thanks for reading! Topics covered by this article
- Section #1 - Short introduction to Django
- Section #2 - Scaffolding a Django Project
- Section #3 - Create a Django new application
- Section #4 - Create a new page template
- Section #5 - Create a new model and use it
- Section #6 - Accommodate with Django Shell
- Section #7 - How to access the admin section
- Free Sample - Argon Dashboard Django
- Free Sample - Django Soft UI Dashboard
- Free Sample - Django Material Dashboard
- Free Sample - Django Volt Dashboard
- Free Sample - Django Black Dashboard
Section #1 - Django Presentation
At the moment this article is published, Django is actively supported by 2k+ contributors with monthly releases and security fixes that keep up the Django core with the latest patterns and concepts used in production. Big tech companies like Instagram
and Disqus
use Django as the main technology for their core services and this argument might be enough to convince beginners to take a look at this amazing web framework. Being in production for more than 15 years, Django community members released in the open-source ecosystem many useful libraries. I will mention just a few below:
- Django Extensions – provides many useful helpers
- Django-environ – manage the environment variables with ease
- Django REST framework – a flexible toolkit for coding APIs fast
- Django-Allauth – a popular module for social logins using Google, Facebook, Github, and many more
To learn more than this article provides, feel free to access the official Django website and documentation.
Section #2 - Scaffolding a Django Project
The goal of this section is to generate a minimal Django starter using the console. before we start, it might be a good idea to check if Python is properly installed and accessible in the terminal.
Step 1# – Check Python version
$ python --version
Python 3.8.4
Step #2 - Create and activate a virtual environment
# Unix based systems
$ virtualenv env
$ source env/bin/activate
Step #3 - Create the project
$ mkdir hellodjango
$ cd hellodjango
Once our working directory is hellodjango
(feel free to use another name), the next step is to call django-admin
and generate the project:
$ django-admin startproject config .
Step #4 - Start the project
$ python manage.py runserver
If all goes well, our newly created Django app should be visible in the browser.
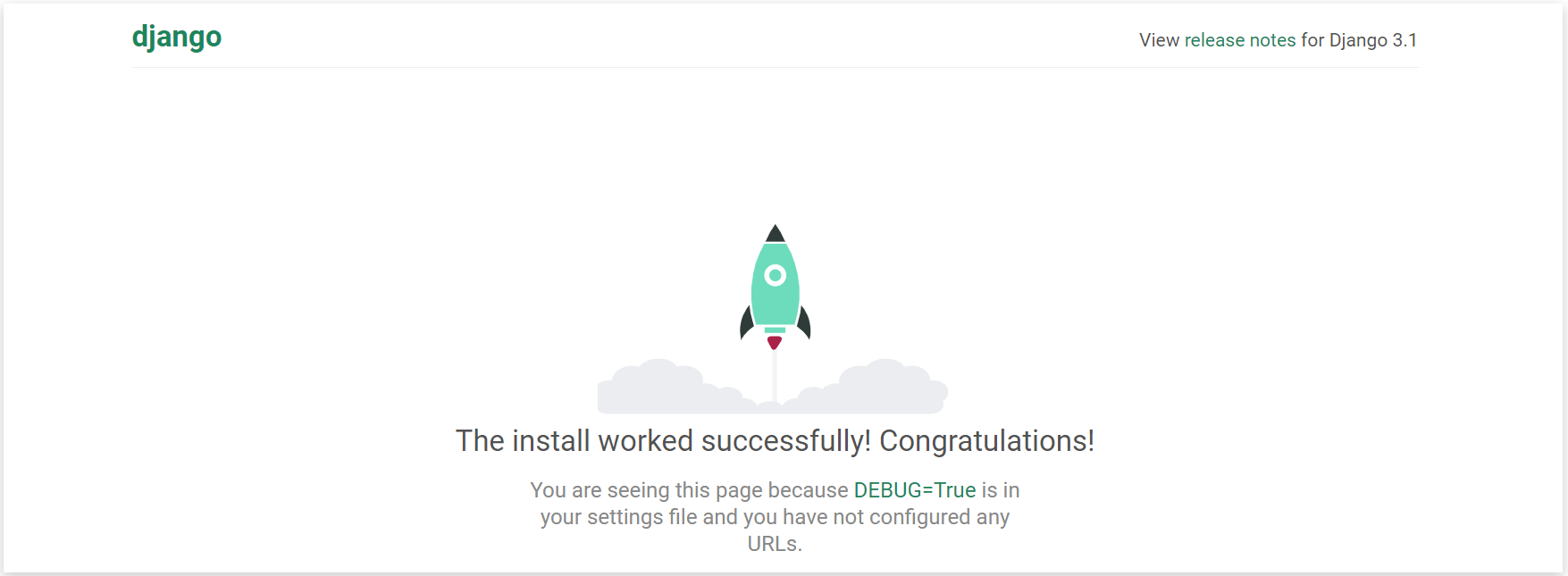
Section #3 - Create a Django App
Django by default has a modular codebase based on apps
. This pattern impacts the project maintenance and evolutions in a positive way because features are isolated and test-able
. When a legacy project needs to be enhanced with a new core feature we might use a new app
for this and the necessary steps are listed below:
- Navigate to the root project folder
- Create the
app
running the command$python manage.py startapp <new_app_name>
- Inform Django that a new “app” is registered (this is not done automatically) by adding “app name” to
INSTALLED_APPS
section in the core settings file - Execute Django migration and we are good to go with the new
app
$ python manage.py migrate
$ python manage.py runserver
Section #4 - Create a new Template Page
Using templates
in our projects is something that helps us to win time and reuse components when new pages are built. Django comes with an intuitive structure regarding this topic as shown below:

Once the template is defined, we can serve the file to users:

Section #5 - Create a new Model
In Django world, a model stands for a new table required by our project to save new information. Please take a look at the necessary steps to define and use a new model:
1# – Update the models.py
file saved in the app
directory with the new table definition
from django.db import models
class Employee(models.Model):
first_name = models.CharField(max_length=30)
last_name = models.CharField(max_length=30)
role = models.CharField(max_length=10)
2# – Generate the SQL code and effectively add the table to the database:
$ python manage.py makemigrations test_app
$ python manage.py migrate
At this point, we can use the new model in our project.
Section #6 - Django Shell
This powerful feature allows us to interact with project code, inspect classes, call helpers and query the database.
$ python manage.py shell
The above command typed in the root of any Django project will open the interactive shell
and we do many things with ease. To see this in action, let’s select all registered users.
$ python manage.py shell
>>> from django.contrib.auth.models import User
>>> all_users = User.objects.all()
In the same manner, we can update the information, a user for instance.
$ python manage.py shell
>>> from django.contrib.auth.models import User
>>> user = User.objects.get(username="testuser")
>>> user.is_admin = True
>>> user.save()
Section #7 - Access ADMIN Section
Django unlocks the administration
section only for superusers. To create a superuser
we should use the Django shell:
$ python manage.py createsuperuser
Once the superuser
is created we can authenticate and manage visually all tables and users defined in the project.
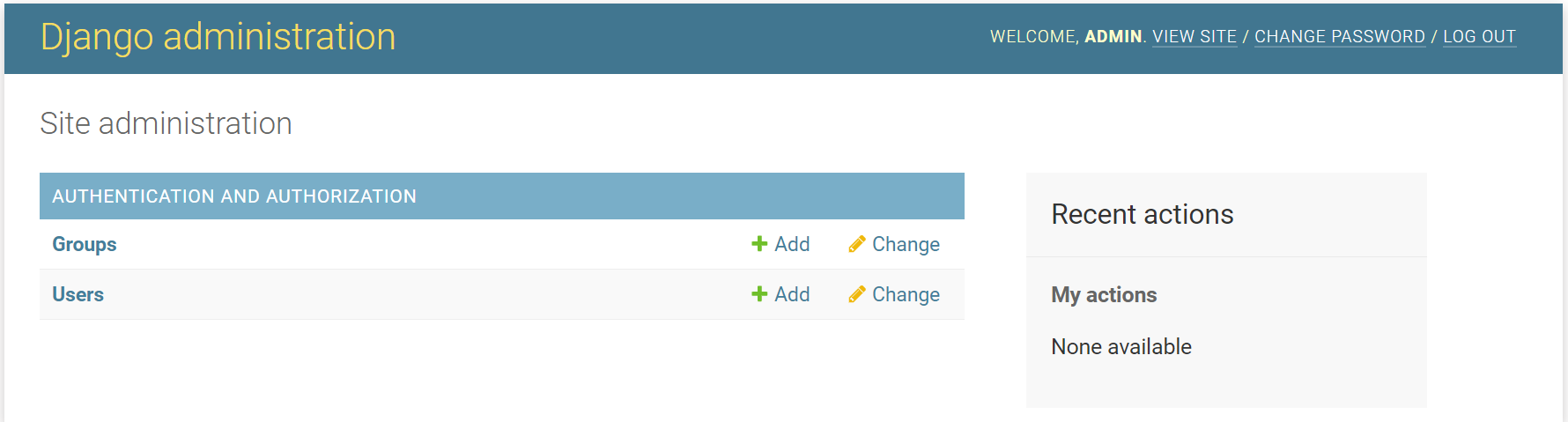
With all this fresh information in mind, it might be a good idea to start coding something useful on top of a few production-ready starters provided by Creative-Tim and AppSeed App Generator. All mentioned projects can be downloaded from Github and the permissive license allows the usage for hobby and commercial projects.
Argon Dashboard Django
This simple starter comes with a few useful features like authentication, database and deployment scripts out-of-the-box
. The product is built on top of Argon Dashboard design (free version) and might be used to bootstrap faster a new project by any developer with basic programming knowledge.
- Argon Dashboard Django - product page (contains DEMO & sources)
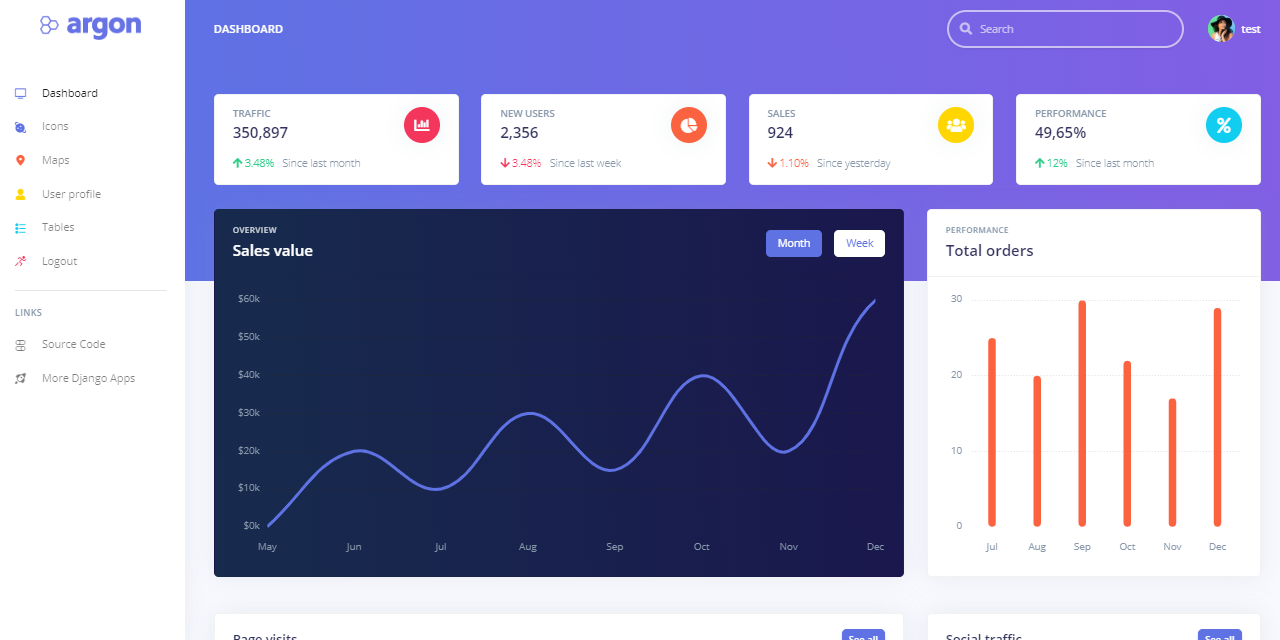
Soft UI Dashboard Django
Admin Dashboard generated by AppSeed in Django Framework. Designed for those who like bold elements and beautiful websites, Soft UI Dashboard is ready to help you create stunning websites and web apps. Soft UI Dashboard is built with over 70 frontend individual elements, like buttons, inputs, navbars, nav tabs, cards, or alerts, giving you the freedom of choosing and combining.
- Soft UI Dashboard Django - product page (contains DEMO & sources)
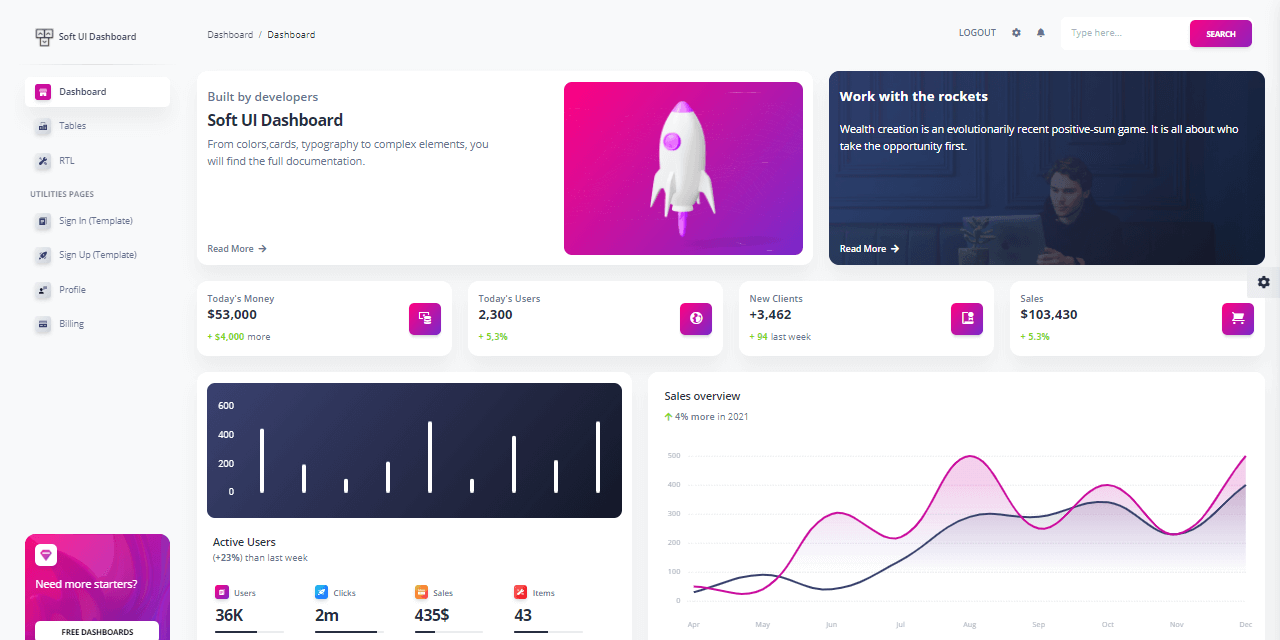
Material Dashboard Django
Start your development with a beautiful Material Admin template for Django. Material Dashboard makes use of light, surface, and movement. The general layout resembles sheets of paper following multiple different layers so that the depth and order is obvious. The navigation stays mainly on the left sidebar and the content is on the right inside the main panel.
- Material Dashboard Django - product page (contains DEMO & sources)
Django codebase is crafted using a simple, modular structure that follows the best practices and provides authentication, database configuration, and deployment scripts for Docker, a popular virtualization software.
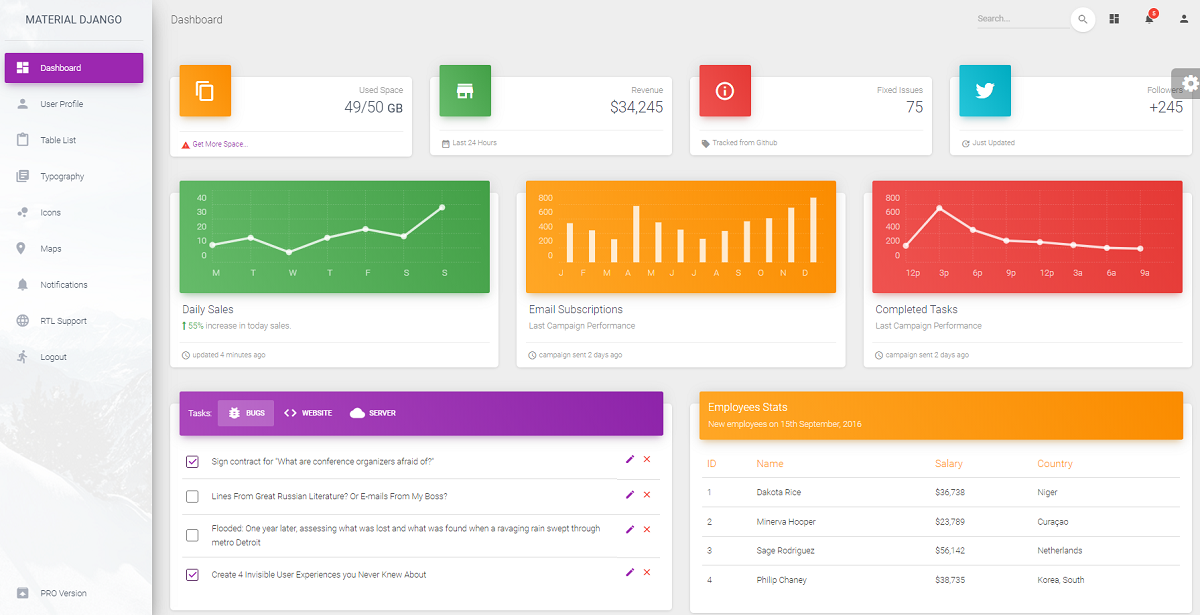
Django Volt Bootstrap 5
Admin Dashboard generated by AppSeed in Django Framework. Volt Dashboard is a free and open-source
Bootstrap 5 Admin Dashboard featuring over 100 components, 11 example pages, and 3 plugins with Vanilla JS. There is more than 100 free Bootstrap 5 components included some of them being buttons, alerts, modals, date pickers, and so on.
- Django Volt Bootstrap 5 - product page (contains DEMO & sources)
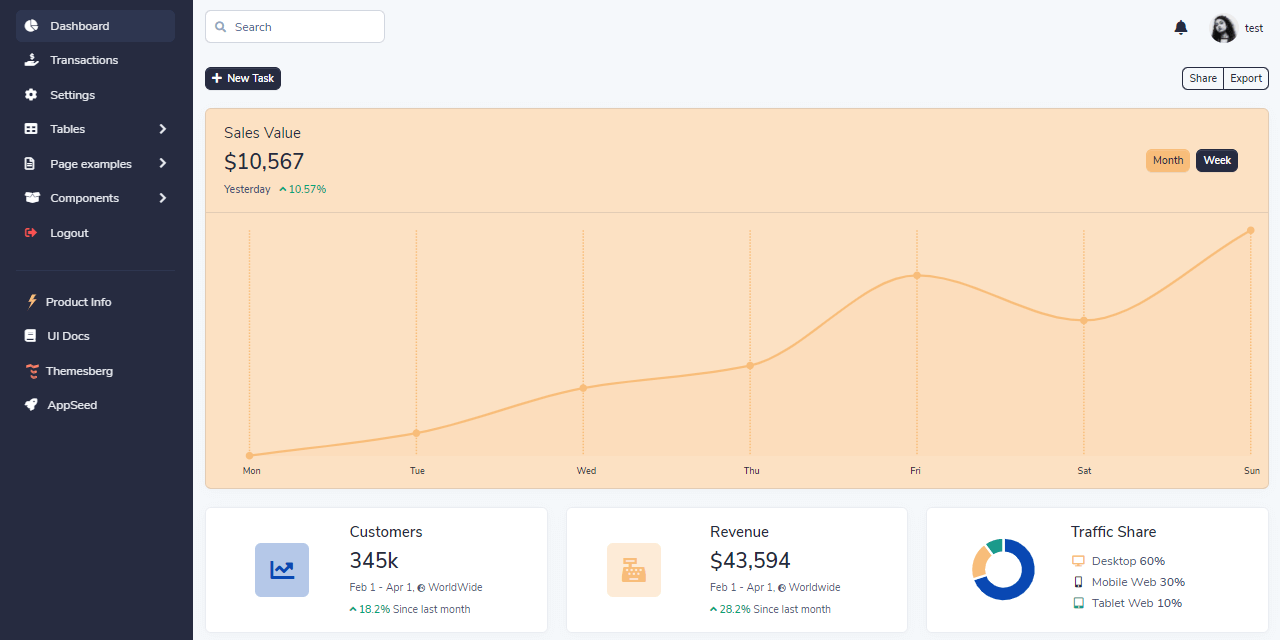
Black Dashboard Django
Creative Tim partnered with AppSeed App Generator to provide better products for developers and designers. Our beautiful UI Kits are integrated into production-ready starters enhanced with database, authentication, modular codebase, and deployment scripts for well-known configurations like Docker, Gunicorn/Nginx.
- Black Dashboard Django - product page (contains DEMO & sources)
Django codebase is crafted using a simple, modular structure that follows the best practices and provides authentication, database configuration, and deployment scripts for Docker, a popular virtualization software. Any developer with basic Django/Python knowledge, by following the product documentation should be able to compile and use the app by typing only a few lines in the terminal.
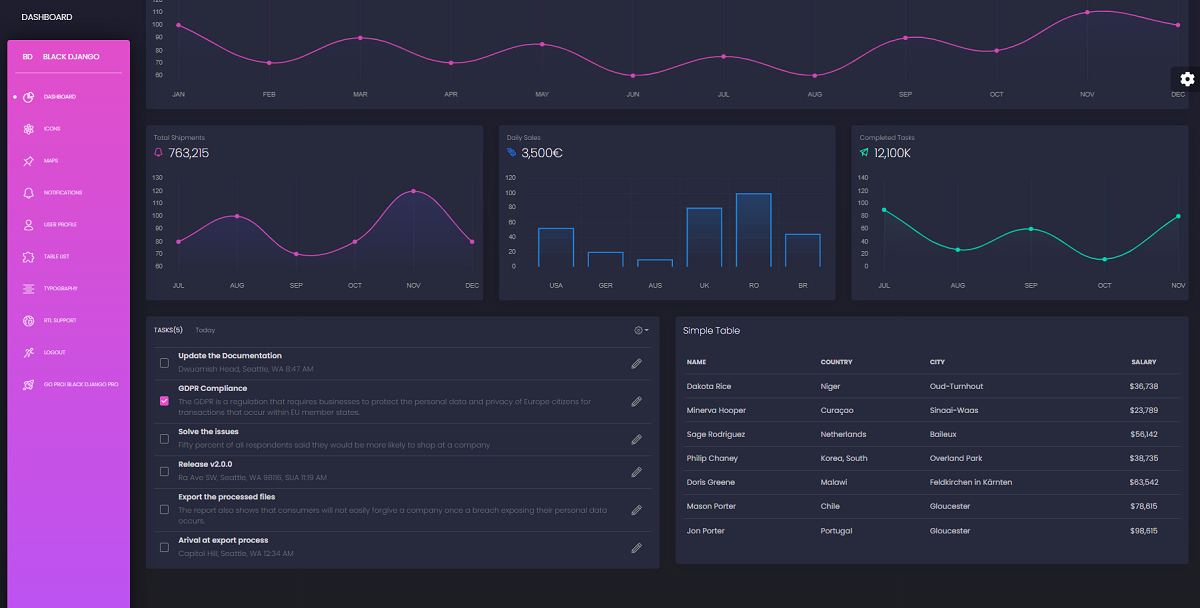
Thank you for reading! For more resources, please access:
- A curated index with more Django Templates provided by Creative-Tim
- More Django Dashboards provided by App Generator